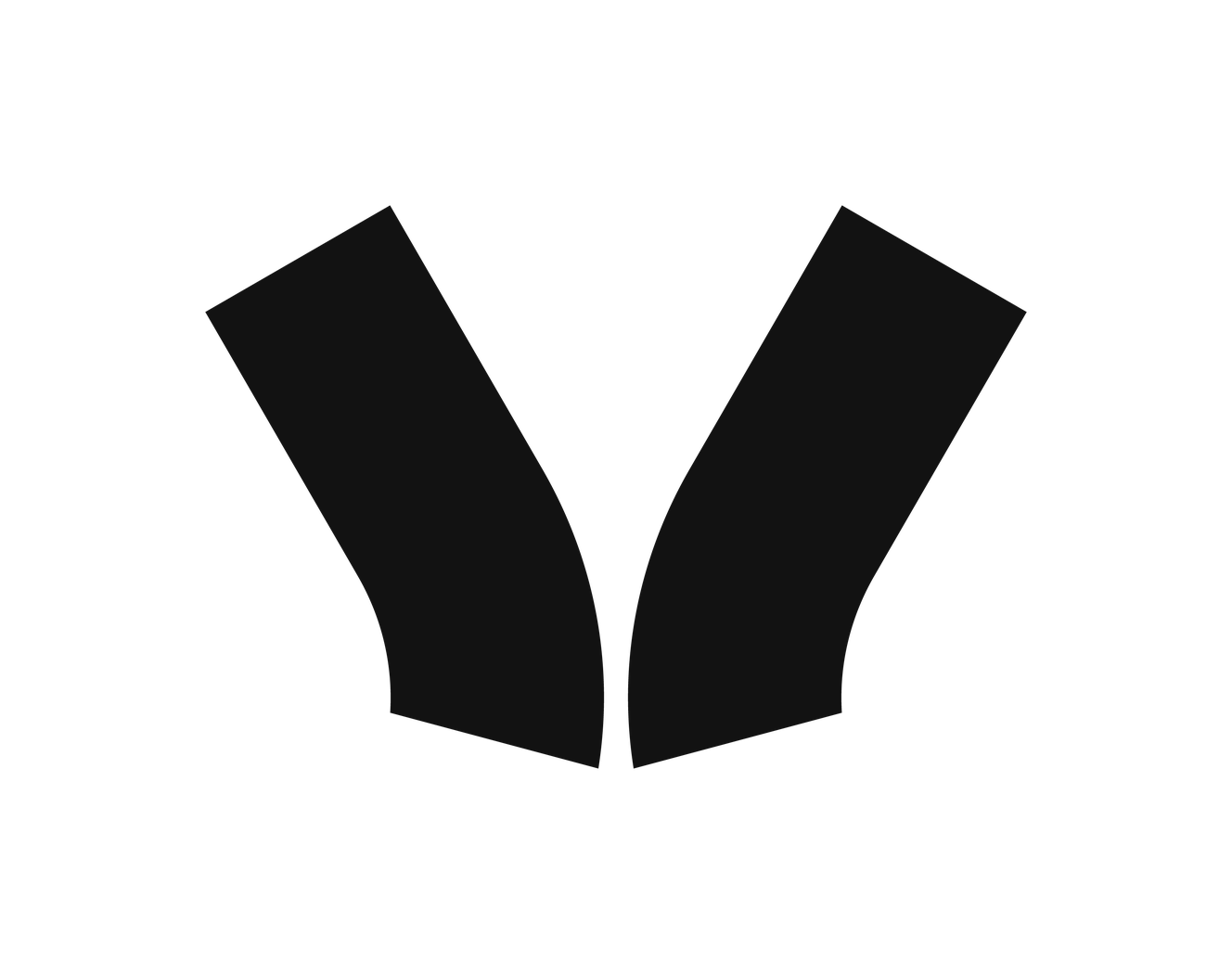 |
Wirepas SDK
|
|
Go to the documentation of this file.
13 #ifndef BOARD_PCA10112_BOARD_H_
14 #define BOARD_PCA10112_BOARD_H_
77 #define BOARD_USART_TX_PIN 6
78 #define BOARD_USART_RX_PIN 8
79 #define BOARD_USART_CTS_PIN 7
80 #define BOARD_USART_RTS_PIN 5
83 #define BOARD_GPIO_PIN_LIST {13, \
94 #define BOARD_GPIO_ID_LED1 0 // mapped to pin P0.13
95 #define BOARD_GPIO_ID_LED2 1 // mapped to pin P0.14
96 #define BOARD_GPIO_ID_LED3 2 // mapped to pin P0.15
97 #define BOARD_GPIO_ID_LED4 3 // mapped to pin P0.16
98 #define BOARD_GPIO_ID_BUTTON1 4 // mapped to pin P0.11
99 #define BOARD_GPIO_ID_BUTTON2 5 // mapped to pin P0.12
100 #define BOARD_GPIO_ID_BUTTON3 6 // mapped to pin P0.24
101 #define BOARD_GPIO_ID_BUTTON4 7 // mapped to pin P0.25
102 #define BOARD_GPIO_ID_USART_WAKEUP 8 // mapped to pin P0.08
105 #define BOARD_LED_ID_LIST {BOARD_GPIO_ID_LED1, BOARD_GPIO_ID_LED2, BOARD_GPIO_ID_LED3, BOARD_GPIO_ID_LED4}
108 #define BOARD_LED_ACTIVE_LOW true
111 #define BOARD_BUTTON_ID_LIST {BOARD_GPIO_ID_BUTTON1, BOARD_GPIO_ID_BUTTON2, BOARD_GPIO_ID_BUTTON3, BOARD_GPIO_ID_BUTTON4}
114 #define BOARD_BUTTON_ACTIVE_LOW true
119 #ifdef BOARD_SUPPORT_DCDC
120 #error This option has been moved to board/<board_name>/config.mk
124 #define BOARD_FEM_MODE_PIN 17 // P0.17
125 #define BOARD_FEM_RXEN_PIN 19 // P0.19
126 #define BOARD_FEM_ANTSEL_PIN 20 // P0.20
127 #define BOARD_FEM_CS_PIN 21 // P0.21
128 #define BOARD_FEM_TXEN_PIN 22 // P0.22
129 #define BOARD_FEM_PDN_PIN 23 // P0.23
132 #define BOARD_FEM_SPI_MOSI_PIN 45 // P1.13
133 #define BOARD_FEM_SPI_MISO_PIN 46 // P1.14
134 #define BOARD_FEM_SPI_CLK_PIN 47 // P1.15