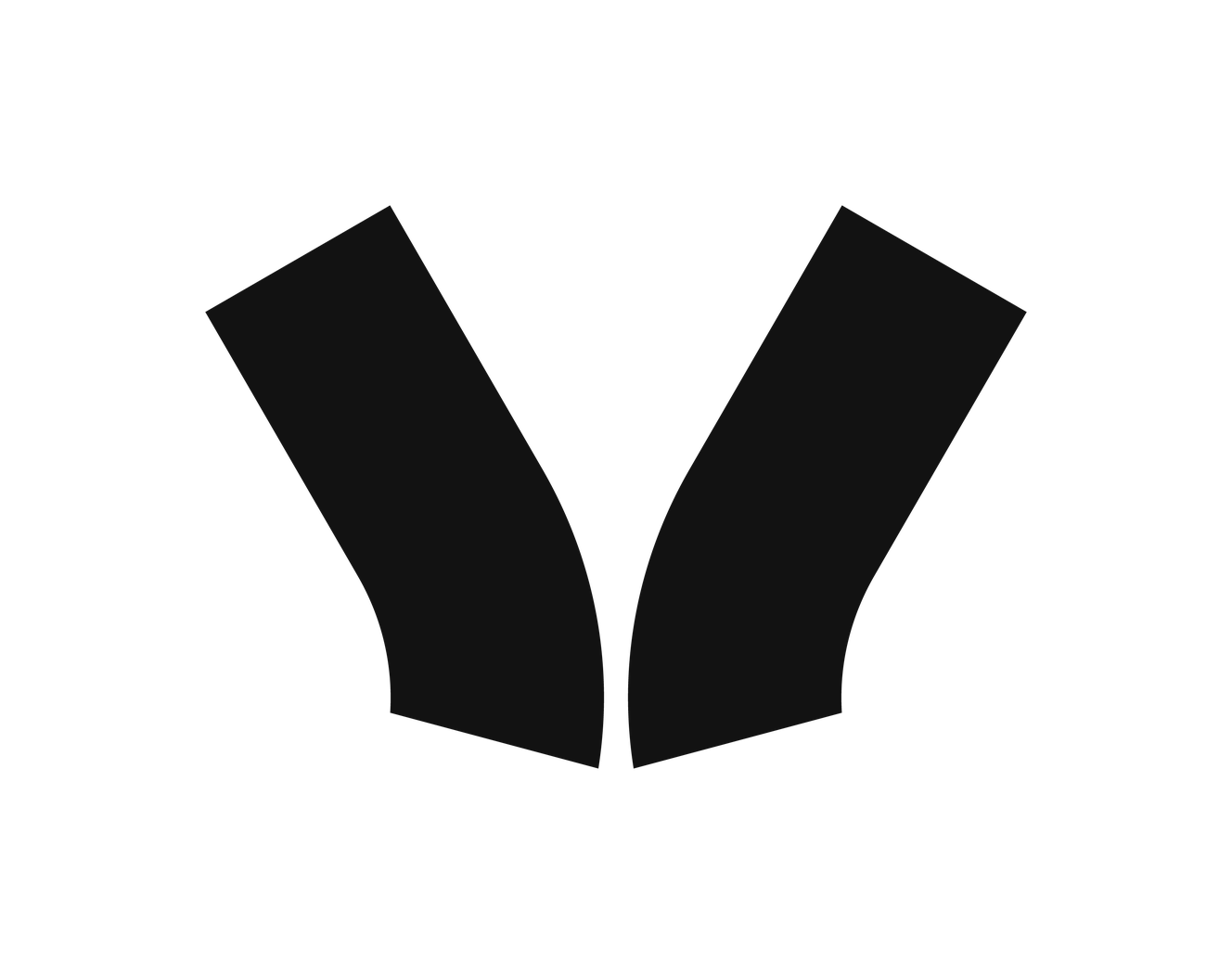 |
Wirepas SDK
|
|
Go to the documentation of this file.
7 #ifndef APP_WAPSUART_H__
8 #define APP_WAPSUART_H__
void Waps_uart_AutoPowerOff(void)
Disable automatic RX power.
void Waps_uart_powerOff(void)
Grafecully power down UART, if autopower-feature is used.
uint32_t Waps_uart_powerExec(void)
Callback task for power manager.
void Waps_uart_powerReset(void)
Power config. Disable UART auto-powering on Sinks and LL Nodes.
void Waps_uart_clean(void)
Clean waps from old data.
void Waps_uart_AutoPowerOn(void)
Enable automatic RX power.
bool(* new_frame_cb_f)(void *, uint32_t)
bool Waps_uart_send(const void *buffer, uint32_t size)
WAPS UART send, send a block of data via serial port Automatically applies SLIP encoding to data and ...
void Waps_uart_setIrq(bool state)
Set indication that something is pending.
bool Waps_uart_init(new_frame_cb_f frame_cb, uint32_t baud, bool flow_ctrl, void *tx_buffer, void *rx_buffer)
WAPS UART initialize, after this, WAPS UART is ready to transmit and receive serial data.
void Waps_uart_keepPowerOn(void)
Keep power on (receiver is receiving)
void Waps_uart_flush(void)
Flush UART module TX buffer. Waits for operation (pend) to complete before returning.