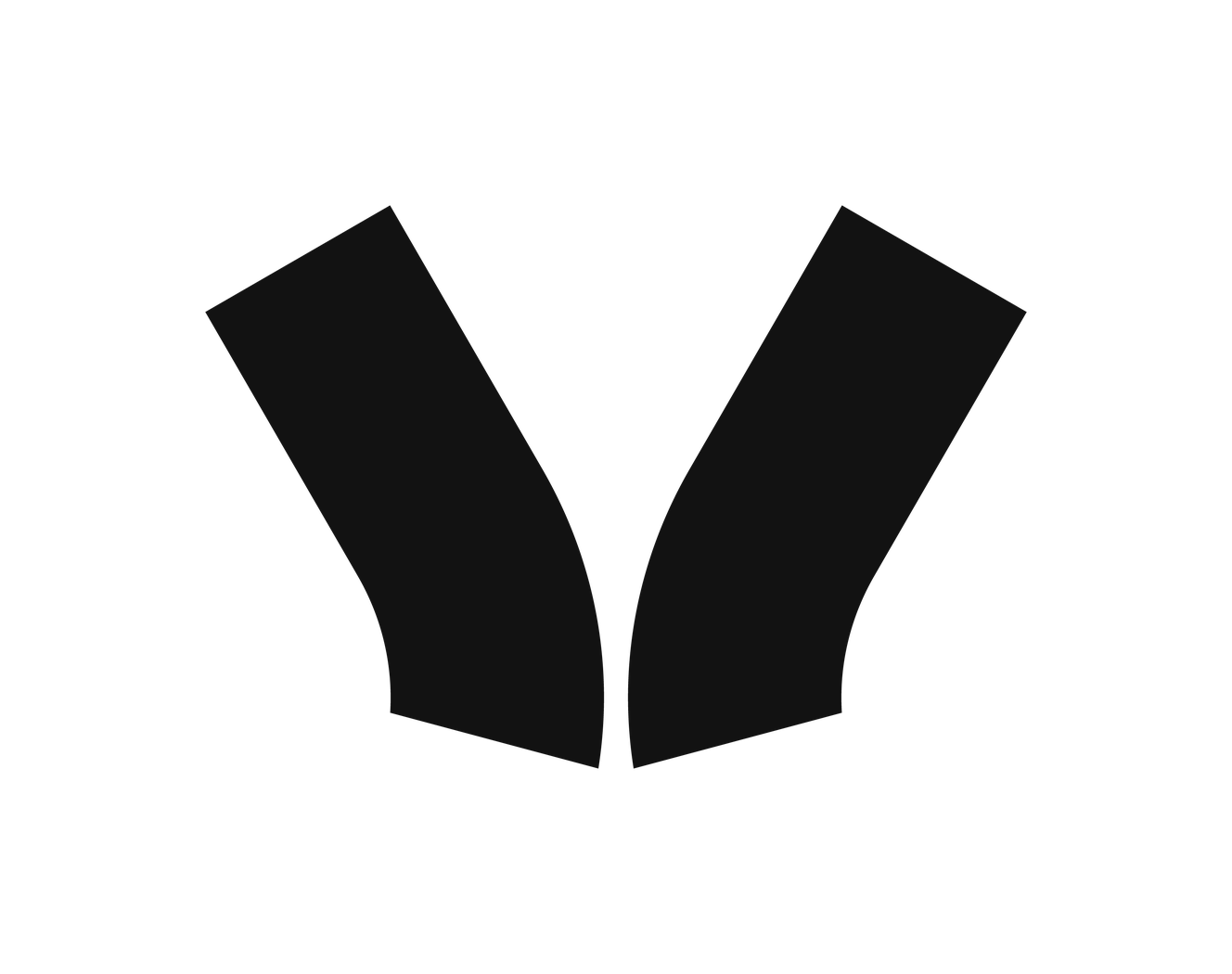 |
Wirepas SDK
|
|
Go to the documentation of this file.
13 #ifndef BOARD_SILABS_BRD4210A_BOARD_H_
14 #define BOARD_SILABS_BRD4210A_BOARD_H_
17 #define BOARD_USART_ID 0
18 #define BOARD_USART_TX_PORT GPIO_PORTA
19 #define BOARD_USART_TX_PIN 8
20 #define BOARD_USART_RX_PORT GPIO_PORTA
21 #define BOARD_USART_RX_PIN 9
24 #define BOARD_GPIO_PIN_LIST {{GPIO_PORTB, 2}, \
33 #define BOARD_GPIO_ID_LED0 0 // mapped to pin PB02
34 #define BOARD_GPIO_ID_LED1 1 // mapped to pin PD03
35 #define BOARD_GPIO_ID_BUTTON0 2 // mapped to pin PB01
36 #define BOARD_GPIO_ID_BUTTON1 3 // mapped to pin PB03
37 #define BOARD_GPIO_ID_USART_WAKEUP 4 // mapped to pin PA09
38 #define BOARD_GPIO_ID_VCOM_ENABLE 5 // mapped to pin PB00
39 #define BOARD_GPIO_ID_SPI_CS 6 // mapped to pin PC04
42 #define BOARD_LED_ID_LIST {BOARD_GPIO_ID_LED0, BOARD_GPIO_ID_LED1}
45 #define BOARD_LED_ACTIVE_LOW false
48 #define BOARD_BUTTON_ID_LIST {BOARD_GPIO_ID_BUTTON0, BOARD_GPIO_ID_BUTTON1}
51 #define BOARD_BUTTON_ACTIVE_LOW true
54 #define BOARD_BUTTON_INTERNAL_PULL false
58 #define BOARD_SPI USART0
59 #define BOARD_SPIROUTE GPIO->USARTROUTE[0]
61 #define BOARD_SPI_EXTFLASH_MOSI_PORT GPIO_PORTC
62 #define BOARD_SPI_EXTFLASH_MISO_PORT GPIO_PORTC
63 #define BOARD_SPI_EXTFLASH_SCKL_PORT GPIO_PORTC
64 #define BOARD_SPI_EXTFLASH_MOSI_PIN 1
65 #define BOARD_SPI_EXTFLASH_MISO_PIN 2
66 #define BOARD_SPI_EXTFLASH_SCKL_PIN 3