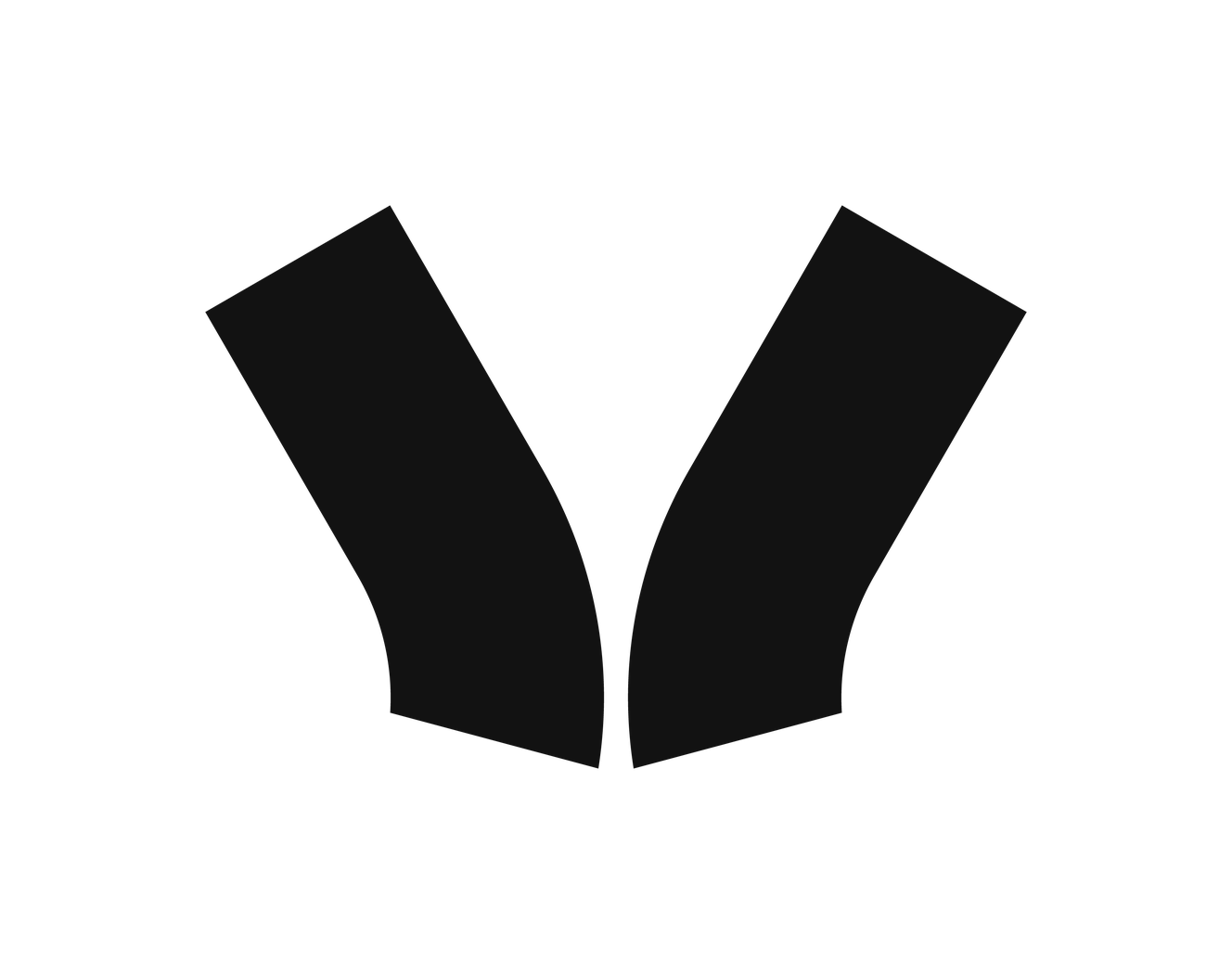 |
Wirepas SDK
|
|
Go to the documentation of this file.
59 #define Print_Log(fmt, ...) UartPrint_printf(fmt, ##__VA_ARGS__)
60 #ifndef DEBUG_LOG_UART_BAUDRATE
61 #define DEBUG_LOG_UART_BAUDRATE 115200
63 #define LOG_INIT() UartPrint_init(DEBUG_LOG_UART_BAUDRATE)
65 #define Print_Log(fmt, ...)
74 #ifndef DEBUG_LOG_MAX_LEVEL
76 #define DEBUG_LOG_MAX_LEVEL LVL_ERROR
91 #define LVL_STRING_4 "D"
92 #define LVL_STRING_3 "I"
93 #define LVL_STRING_2 "W"
94 #define LVL_STRING_1 "E"
95 #define LVL_STRING_0 ""
100 #define DEBUG_LVL_TO_STRING(level) LVL_STRING_##level
105 #define FLUSH_DELAY_MS 45
108 #ifndef DEBUG_LOG_CUSTOM
109 # ifndef DEBUG_LOG_MODULE_NAME
111 # error "No module name set for logger"
114 # define DEBUG_LOG_PRINT_MODULE_NAME
115 # define DEBUG_LOG_PRINT_TIME
116 # define DEBUG_LOG_PRINT_LEVEL
117 # undef DEBUG_LOG_PRINT_FUNCTION
118 # undef DEBUG_LOG_PRINT_LINE
122 #ifdef DEBUG_LOG_PRINT_MODULE_NAME
123 # define S_MOD_NAME_PREFIX "["DEBUG_LOG_MODULE_NAME"]"
125 # define S_MOD_NAME_PREFIX
128 #ifdef DEBUG_LOG_PRINT_TIME_HP
129 # define S_TIME_PREFIX "[%09u]"
130 # define S_TIME_SUFFIX , lib_time->getTimestampHp()
131 #elif defined(DEBUG_LOG_PRINT_TIME)
132 # define S_TIME_PREFIX "[%09u]"
133 # define S_TIME_SUFFIX , lib_time->getTimestampCoarse()
135 # define S_TIME_PREFIX
136 # define S_TIME_SUFFIX
139 #ifdef DEBUG_LOG_PRINT_LEVEL
140 # define S_LEVEL_PREFIX(level) " "DEBUG_LVL_TO_STRING(level)": "
142 # define S_LEVEL_PREFIX(level)
145 #ifdef DEBUG_LOG_PRINT_FUNCTION
146 # define S_FUNCTION_PREFIX "func:%s, "
147 # define S_FUNCTION_SUFFIX , __FUNCTION__
149 # define S_FUNCTION_PREFIX
150 # define S_FUNCTION_SUFFIX
153 #ifdef DEBUG_LOG_PRINT_LINE
154 # define S_LINE_PREFIX "line: %03d, "
155 # define S_LINE_SUFFIX , __LINE__
157 # define S_LINE_PREFIX
158 # define S_LINE_SUFFIX
172 #define LOG(level, fmt, ...) \
174 ((uint8_t) level) <= ((uint8_t) DEBUG_LOG_MAX_LEVEL) ? \
176 S_MOD_NAME_PREFIX S_TIME_PREFIX S_LEVEL_PREFIX(level) \
177 S_FUNCTION_PREFIX S_LINE_PREFIX \
178 fmt"\n" S_TIME_SUFFIX S_FUNCTION_SUFFIX S_LINE_SUFFIX \
193 #define LOG_BUFFER(level, buffer, size) \
195 if(((uint8_t) level) <= ((uint8_t) DEBUG_LOG_MAX_LEVEL)) { \
196 for (uint8_t i = 0; i < size; i++) \
198 Print_Log("%02X ", buffer[i]); \
199 if ((i & 0xF) == 0xF && i != (uint8_t)(size-1)) \
213 #define LOG_FLUSH(level) \
215 if(((uint8_t) level) <= ((uint8_t) DEBUG_LOG_MAX_LEVEL)) { \
216 app_lib_time_timestamp_hp_t end; \
217 end = lib_time->addUsToHpTimestamp(lib_time->getTimestampHp(), \
218 FLUSH_DELAY_MS * 1000); \
219 while (lib_time->isHpTimestampBefore(lib_time->getTimestampHp(),end)); \