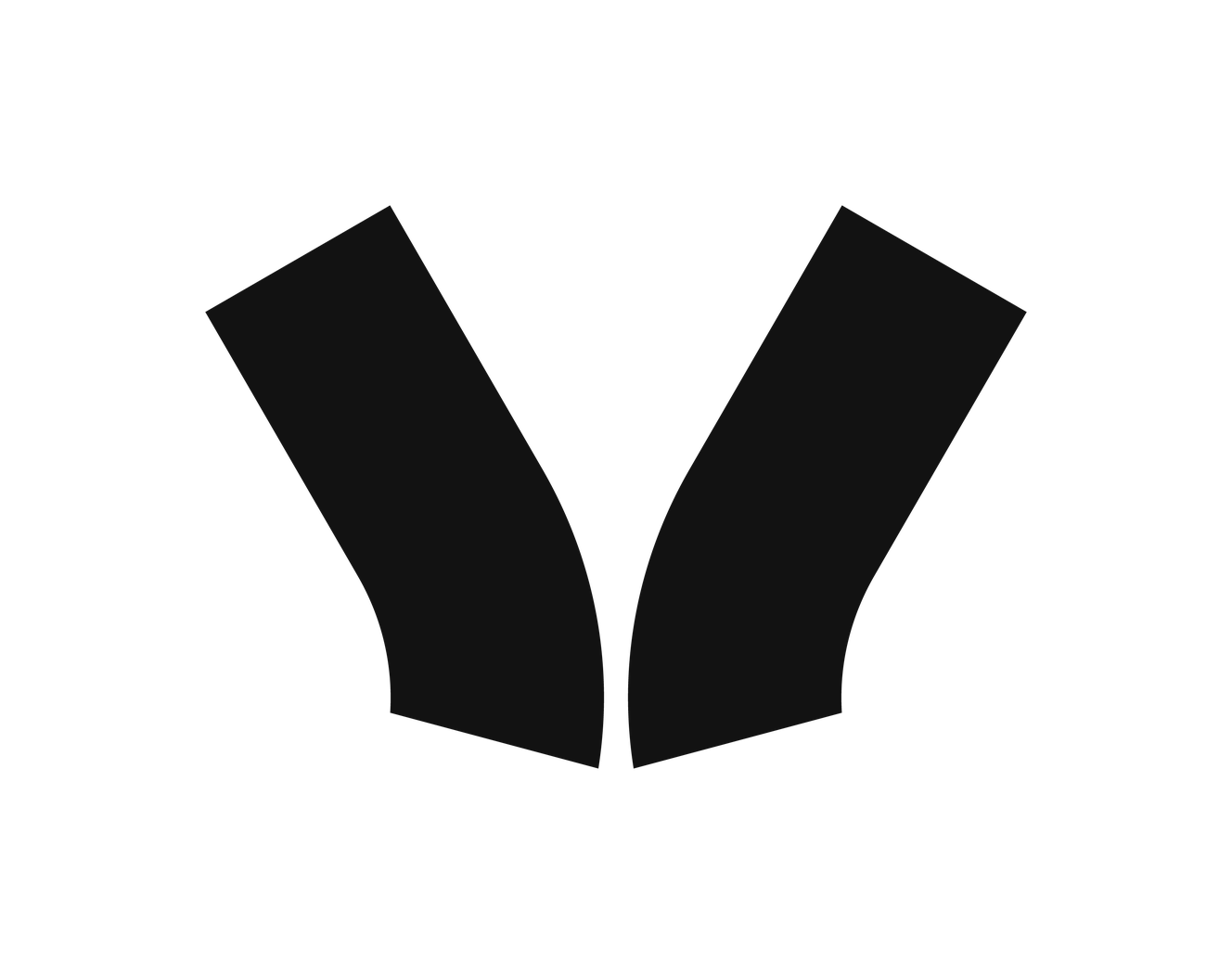 |
Wirepas SDK
|
|
void Usart_receiverOn(void)
Enable USART Receiver.
void Usart_setEnabled(bool enabled)
Enable or disable USART.
void Usart_enableReceiver(serial_rx_callback_f callback)
Enable UART receiver Set callback handlers for character RX event Enables interrupt source and event,...
void Usart_flush(void)
Soft flush (dump data to I/O) UART TX buffer.
bool Usart_setFlowControl(uart_flow_control_e flow)
Set USART flow control mode.
void Usart_receiverOff(void)
Disable USART Receiver.
uint32_t Usart_getMTUSize(void)
Returns the maximum transmission unit in bytes for the USART (= maximum length of a single message)
void Usart_init(uint32_t baudrate)
Initialize all USART blocks Enables peripheral clocks and disables peripherals for the duration of th...
uint32_t Usart_sendBuffer(const void *buf, uint32_t len)
Send a buffer to USART.
void(* serial_rx_callback_f)(uint8_t *ch, size_t n)