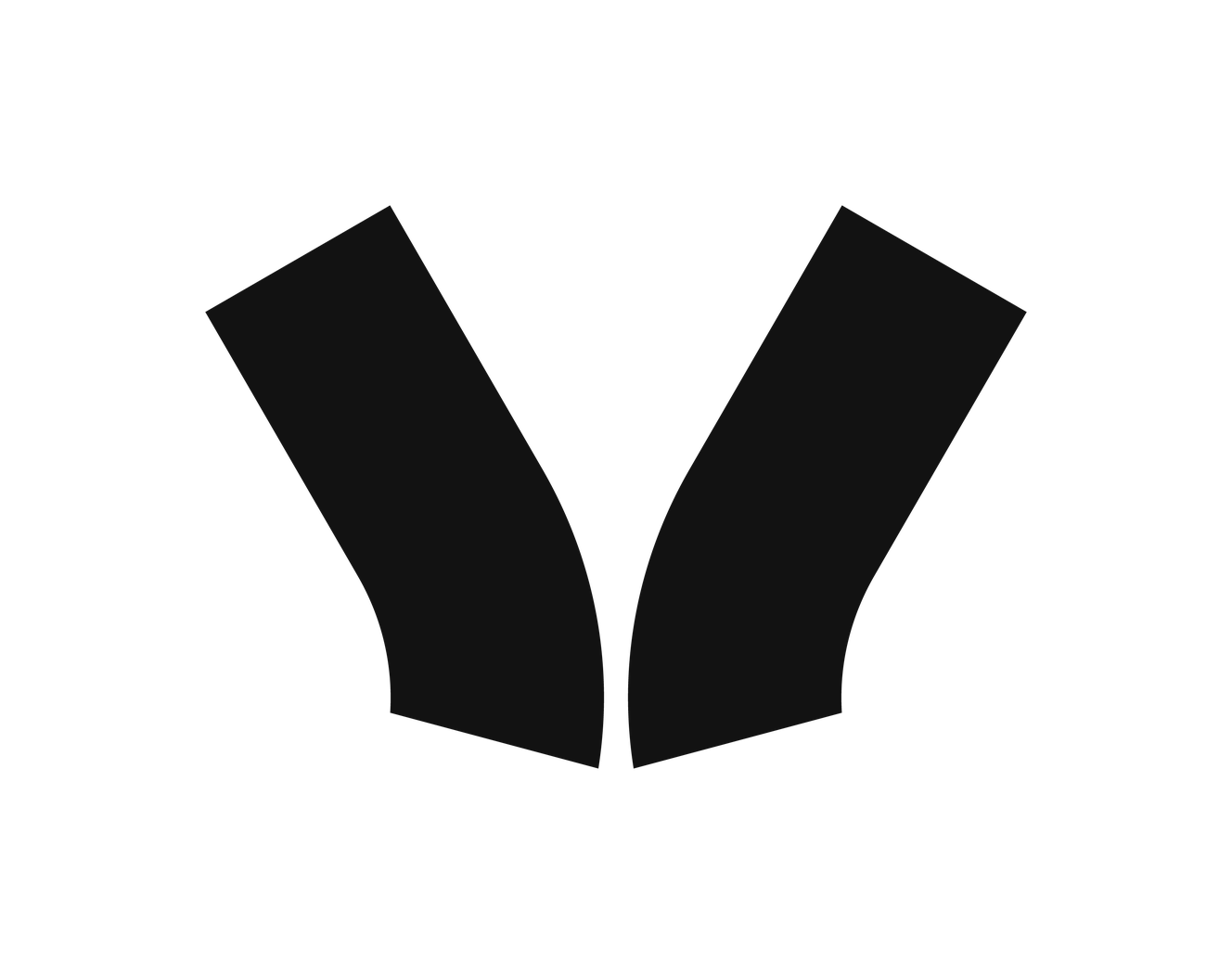 |
Wirepas SDK
|
|
Go to the documentation of this file.
18 #define APP_CONF_MAX 80
33 #define MSAP_MAX_NBORS 8
36 #define MSAP_AUTOSTART 1
54 typedef struct __attribute__ ((__packed__))
114 MULTICAST_ADDRESS_AMOUNT *
sizeof(
w_addr_t),
120 typedef struct __attribute__ ((__packed__))
125 typedef struct __attribute__ ((__packed__))
134 typedef struct __attribute__ ((__packed__))
142 typedef struct __attribute__ ((__packed__))
148 typedef struct __attribute__ ((__packed__))
156 typedef struct __attribute__ ((__packed__))
166 typedef struct __attribute__ ((__packed__))
176 typedef struct __attribute__ ((__packed__))
193 typedef struct __attribute__ ((__packed__))
217 #define MSAP_SCRATCHPAD_BLOCK_MAX_NUM_BYTES 112
220 typedef struct __attribute__ ((__packed__))
230 #define FRAME_MSAP_SCRATCHPAD_BLOCK_REQ_HEADER_SIZE \
231 (sizeof(msap_scratchpad_block_req_t) - MSAP_SCRATCHPAD_BLOCK_MAX_NUM_BYTES)
255 typedef struct __attribute__ ((__packed__))
288 typedef struct __attribute__ ((__packed__))
304 typedef struct __attribute__ ((__packed__))
346 typedef struct __attribute__ ((__packed__))
353 typedef struct __attribute__ ((__packed__))
372 typedef struct __attribute__ ((__packed__))
381 typedef struct __attribute__ ((__packed__))
390 typedef struct __attribute__ ((__packed__))
426 typedef struct __attribute__ ((__packed__))
452 typedef struct __attribute__ ((__packed__))
464 #define MSAP_SCRATCHPAD_BLOCK_READ_MAX_NUM_BYTES (MSAP_SCRATCHPAD_BLOCK_MAX_NUM_BYTES)
467 typedef struct __attribute__ ((__packed__))
493 typedef struct __attribute__ ((__packed__))
501 #define FRAME_MSAP_SCRATCHPAD_BLOCK_READ_CNF_HEADER_SIZE \
502 (sizeof(msap_scratchpad_block_read_cnf_t) - MSAP_SCRATCHPAD_BLOCK_READ_MAX_NUM_BYTES)
@ MSAP_SCRATCHPAD_TARGET_INVALID_ROLE
msap_neighbor_type_e
MSAP nbor type.
@ MSAP_SCRATCHPAD_BLOCK_READ_INVALID_START_ADDR
uint32_t processed_num_bytes
@ MSAP_SLEEP_INVALID_VALUE
@ MSAP_SCRATCHPAD_START_ACCESS_DENIED
@ MSAP_ATTR_SCRATCHPAD_BLOCK_MAX
msap_remote_status_ind_t remote_status_ind
uint16_t update_req_timeout
msap_scratchpad_block_read_req_t scratchpad_block_read_req
msap_remote_status_req_t remote_status_req
@ MSAP_SCRATCHPAD_START_SUCCESS
@ MSAP_SCRATCHPAD_BLOCK_READ_ACCESS_DENIED
@ MSAP_ATTR_RESERVED_1_SIZE
@ MSAP_NRLS_SLEEP_NOT_STARTED
msap_scratchpad_target_write_res_e
@ MSAP_SCRATCHPAD_BLOCK_INVALID_NUM_BYTES
@ MSAP_SLEEP_INVALID_STATE
@ MSAP_ATTR_AUTOSTART_SIZE
msap_int_write_req_t int_write_req
msap_scratchpad_target_read_cnf_t scratchpad_target_read_cnf
@ MSAP_SCRATCHPAD_BLOCK_INVALID_DATA
uint8_t otap_seq_t
Type for OTAP sequence number.
msap_install_quality_cnf_t inst_qual_cnf
@ MSAP_SCRATCHPAD_ACTION_LEGACY
#define MSAP_SCRATCHPAD_BLOCK_READ_MAX_NUM_BYTES
@ MSAP_SCRATCHPAD_TARGET_INVALID_VALUE
msap_neighbors_cnf_t nbor_cnf
msap_scratchpad_target_write_req_t scratchpad_target_write_req
@ MSAP_SCRATCHPAD_START_INVALID_STATE
@ MSAP_SCAN_NBORS_INVALID_STATE
#define MSAP_SCRATCHPAD_BLOCK_MAX_NUM_BYTES
uint32_t gotoNRSL_seconds
@ MSAP_ATTR_SCRATCHPAD_BLOCK_MAX_SIZE
@ MSAP_ATTR_PDU_BUFF_USAGE
@ MSAP_SCRATCHPAD_ACTION_NO_OTAP
@ MSAP_SCRATCHPAD_TARGET_ACCESS_DENIED
@ MSAP_ATTR_CURRENT_AC_SIZE
@ MSAP_SCRATCHPAD_BOOTABLE_INVALID_STATE
@ MSAP_SLEEP_ACCESS_DENIED
@ MSAP_SCRATCHPAD_BLOCK_COMPLETED_ERROR
@ MSAP_SCRATCHPAD_BLOCK_NOT_ONGOING
@ MSAP_SCRATCHPAD_BLOCK_READ_INVALID_STATE
@ MSAP_SCRATCHPAD_ACTION_PROPAGATE_ONLY
msap_scratchpad_status_cnf_t scratchpad_status_cnf
@ MSAP_SCRATCHPAD_BOOTABLE_NO_SCRATCHPAD
@ MSAP_ATTR_SCRATCHPAD_NUM_BYTES_SIZE
msap_scratchpad_block_req_t scratchpad_block_req
@ MSAP_SCRATCHPAD_BLOCK_INVALID_STATE
@ MSAP_SCAN_NBORS_SUCCESS
@ MSAP_SCRATCHPAD_START_INVALID_NUM_BYTES
@ MSAP_SCRATCHPAD_BLOCK_COMPLETED_OK
@ MSAP_SCRATCHPAD_TARGET_SUCCESS
#define APP_CONF_MAX
App config max size. The define is needed for compiling time size reservations.
msap_scratchpad_block_read_e
msap_ind_poll_cnf_t ind_poll_cnf
@ MSAP_SCRATCHPAD_BLOCK_INVALID_START_ADDR
@ MSAP_ATTR_PDU_BUFF_CAPA
msap_sink_cost_write_req_t cost_write_req
@ MSAP_ATTR_SCRATCHPAD_NUM_BYTES
@ MSAP_SCRATCHPAD_START_INVALID_SEQ
uint8_t queued_indications
@ MSAP_SCAN_NBORS_ACCESS_DENIED
msap_state_ind_t state_ind
@ MSAP_ATTR_ROUTE_COUNT_SIZE
@ MSAP_SCRATCHPAD_CLEAR_SUCCESS
@ MSAP_SCRATCHPAD_BLOCK_READ_INVALID_NUM_BYTES
@ MSAP_ATTR_PDU_BUFF_CAP_SIZE
msap_scratchpad_block_read_cnf_t scratchpad_block_read_cnf
@ MSAP_ATTR_SYSTEM_TIME_SIZE
@ MSAP_SCRATCHPAD_CLEAR_ACCESS_DENIED
@ MSAP_ATTR_AC_RANGE_SIZE
@ MSAP_SCRATCHPAD_BOOTABLE_SUCCESS
@ MSAP_ATTR_NBOR_COUNT_SIZE
msap_sleep_start_req_t sleep_start_req
@ MSAP_REMOTE_STATUS_ACCESS_DENIED
@ MSAP_ATTR_MCAST_GROUPS_SIZE
@ MSAP_SCRATCHPAD_ACTION_PROPAGATE_AND_PROCESS
msap_scratchpad_bootable_result_e
msap_sleep_state_rsp_t sleep_state_rsp
@ MSAP_SCRATCHPAD_BLOCK_READ_SUCCESS
msap_sink_cost_read_cnf_t cost_read_cnf
msap_sleep_latest_gotosleep_rsp_t sleep_gotosleep_rsp_t
@ MSAP_ATTR_PDU_BUFF_USAGE_SIZE
@ MSAP_ATTR_STACK_STATUS_SIZE
@ MSAP_SCRATCHPAD_ACTION_PROPAGATE_AND_PROCESS_WITH_DELAY
@ MSAP_SCRATCHPAD_BLOCK_READ_NO_SCRATCHPAD
msap_scratchpad_start_req_t scratchpad_start_req
msap_int_read_cnf_t int_read_cnf
msap_on_scanned_nbors_ind_t on_scanned_nbors
@ MSAP_SCRATCHPAD_CLEAR_INVALID_STATE
@ MSAP_ATTR_AC_LIMITS_SIZE
@ MSAP_SCRATCHPAD_BLOCK_SUCCESS
msap_start_req_t start_req
@ MSAP_SLEEP_INVALID_ROLE
@ MSAP_SCRATCHPAD_BOOTABLE_ACCESS_DENIED