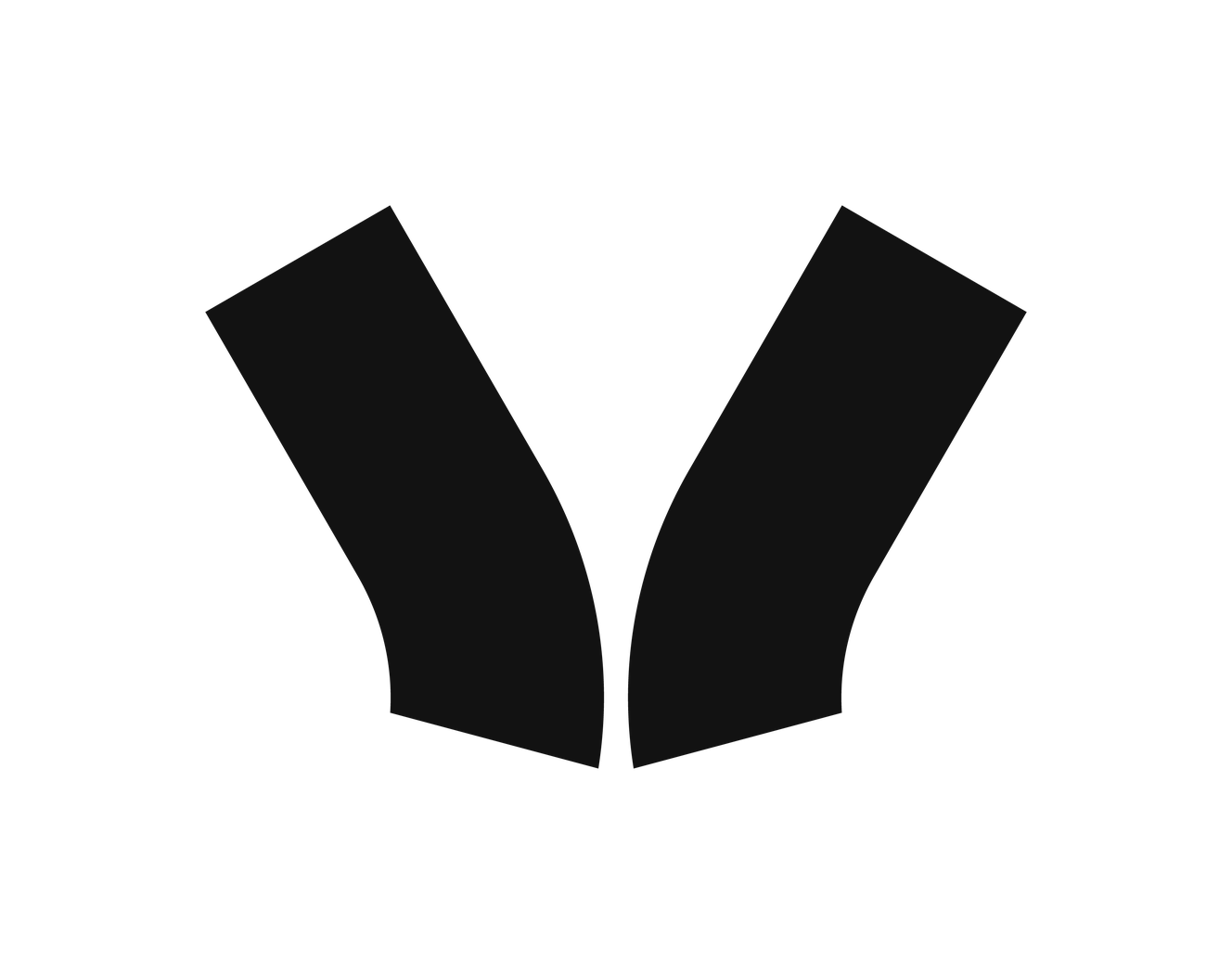 |
Wirepas SDK
|
|
Go to the documentation of this file.
7 #ifndef FUNCTION_CODES_H__
8 #define FUNCTION_CODES_H__
148 #define DSAP_REQUESTS \
150 WAPS_FUNC_DSAP_DATA_TX_REQ, \
151 WAPS_FUNC_DSAP_DATA_TX_TT_REQ, \
152 WAPS_FUNC_DSAP_DATA_TX_FRAG_REQ, \
155 #define MSAP_REQUESTS \
157 WAPS_FUNC_MSAP_INDICATION_POLL_REQ, \
158 WAPS_FUNC_MSAP_STACK_START_REQ, \
159 WAPS_FUNC_MSAP_STACK_STOP_REQ, \
160 WAPS_FUNC_MSAP_ATTR_WRITE_REQ, \
161 WAPS_FUNC_MSAP_ATTR_READ_REQ, \
162 WAPS_FUNC_MSAP_SCRATCHPAD_START_REQ, \
163 WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_REQ, \
164 WAPS_FUNC_MSAP_SCRATCHPAD_STATUS_REQ, \
165 WAPS_FUNC_MSAP_SCRATCHPAD_BOOTABLE_REQ, \
166 WAPS_FUNC_MSAP_SCRATCHPAD_CLEAR_REQ, \
167 WAPS_FUNC_MSAP_REMOTE_STATUS_REQ, \
168 WAPS_FUNC_MSAP_GET_NBORS_REQ, \
169 WAPS_FUNC_MSAP_SCAN_NBORS_REQ, \
170 WAPS_FUNC_MSAP_GET_INSTALL_QUALITY_REQ, \
171 WAPS_FUNC_MSAP_SINK_COST_WRITE_REQ, \
172 WAPS_FUNC_MSAP_SINK_COST_READ_REQ, \
173 WAPS_FUNC_MSAP_APP_CONFIG_WRITE_REQ, \
174 WAPS_FUNC_MSAP_APP_CONFIG_READ_REQ, \
175 WAPS_FUNC_MSAP_STACK_SLEEP_REQ, \
176 WAPS_FUNC_MSAP_STACK_SLEEP_STOP_REQ, \
177 WAPS_FUNC_MSAP_STACK_SLEEP_STATE_GET_REQ, \
178 WAPS_FUNC_MSAP_STACK_SLEEP_GOTOSLEEPINFO_REQ, \
179 WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_READ_REQ, \
180 WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_WRITE_REQ, \
181 WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_READ_REQ \
184 #define CSAP_REQUESTS \
186 WAPS_FUNC_CSAP_ATTR_WRITE_REQ, \
187 WAPS_FUNC_CSAP_ATTR_READ_REQ, \
188 WAPS_FUNC_CSAP_FACTORY_RESET_REQ, \
191 #define WAPS_CONFIRMATIONS \
193 WAPS_FUNC_DSAP_DATA_TX_CNF, \
194 WAPS_FUNC_DSAP_DATA_TX_FRAG_CNF, \
195 WAPS_FUNC_MSAP_INDICATION_POLL_CNF, \
196 WAPS_FUNC_MSAP_STACK_START_CNF, \
197 WAPS_FUNC_MSAP_STACK_STOP_CNF, \
198 WAPS_FUNC_MSAP_APP_CONFIG_WRITE_CNF, \
199 WAPS_FUNC_MSAP_APP_CONFIG_READ_CNF, \
200 WAPS_FUNC_MSAP_ATTR_WRITE_CNF, \
201 WAPS_FUNC_MSAP_ATTR_READ_CNF, \
202 WAPS_FUNC_MSAP_GET_NBORS_CNF, \
203 WAPS_FUNC_MSAP_SCAN_NBORS_CNF, \
204 WAPS_FUNC_MSAP_GET_INSTALL_QUALITY_CNF, \
205 WAPS_FUNC_MSAP_SINK_COST_WRITE_CNF, \
206 WAPS_FUNC_MSAP_SINK_COST_READ_CNF, \
207 WAPS_FUNC_CSAP_ATTR_WRITE_CNF, \
208 WAPS_FUNC_CSAP_ATTR_READ_CNF, \
209 WAPS_FUNC_CSAP_FACTORY_RESET_CNF, \
210 WAPS_FUNC_MSAP_SCRATCHPAD_START_CNF, \
211 WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_CNF, \
212 WAPS_FUNC_MSAP_SCRATCHPAD_STATUS_CNF, \
213 WAPS_FUNC_MSAP_SCRATCHPAD_BOOTABLE_CNF, \
214 WAPS_FUNC_MSAP_SCRATCHPAD_CLEAR_CNF, \
215 WAPS_FUNC_MSAP_REMOTE_STATUS_CNF, \
216 WAPS_FUNC_DSAP_DATA_TX_TT_CNF, \
217 WAPS_FUNC_MSAP_APP_CONFIG_WRITE_CNF, \
218 WAPS_FUNC_MSAP_APP_CONFIG_READ_CNF, \
219 WAPS_FUNC_MSAP_STACK_SLEEP_REQ_CNF, \
220 WAPS_FUNC_MSAP_STACK_SLEEP_STOP_CNF, \
221 WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_READ_CNF, \
222 WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_WRITE_CNF, \
223 WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_READ_CNF, \
226 #define WAPS_INDICATIONS \
228 WAPS_FUNC_DSAP_DATA_TX_IND, \
229 WAPS_FUNC_DSAP_DATA_RX_FRAG_IND, \
230 WAPS_FUNC_DSAP_DATA_RX_IND, \
231 WAPS_FUNC_MSAP_STACK_STATE_IND, \
232 WAPS_FUNC_MSAP_APP_CONFIG_RX_IND, \
233 WAPS_FUNC_MSAP_REMOTE_STATUS_IND, \
234 WAPS_FUNC_MSAP_SCAN_NBORS_IND, \
237 #define WAPS_RESPONSES \
239 WAPS_FUNC_DSAP_DATA_TX_RSP, \
240 WAPS_FUNC_DSAP_DATA_RX_FRAG_RSP, \
241 WAPS_FUNC_DSAP_DATA_RX_RSP, \
242 WAPS_FUNC_MSAP_STACK_STATE_RSP, \
243 WAPS_FUNC_MSAP_APP_CONFIG_RX_RSP, \
244 WAPS_FUNC_MSAP_REMOTE_STATUS_RSP, \
245 WAPS_FUNC_MSAP_SCAN_NBORS_RSP, \
246 WAPS_FUNC_MSAP_STACK_SLEEP_STATE_GET_RSP, \
247 WAPS_FUNC_MSAP_STACK_SLEEP_GOTOSLEEPINFO_RSP, \
@ WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_WRITE_REQ
@ WAPS_FUNC_DSAP_DATA_TX_CNF
@ WAPS_FUNC_DSAP_DATA_RX_FRAG_IND
@ WAPS_FUNC_MSAP_APP_CONFIG_READ_CNF
@ WAPS_FUNC_DSAP_DATA_TX_FRAG_REQ
@ WAPS_FUNC_RESERVED_REMOTE_API_4_REQ
@ WAPS_FUNC_DSAP_DATA_TX_REQ
@ DEPRECATED_WAPS_FUNC_MSAP_REMOTE_UPDATE_REQ
@ WAPS_FUNC_MSAP_STACK_SLEEP_STATE_GET_RSP
@ WAPS_FUNC_MSAP_SCAN_NBORS_REQ
@ WAPS_FUNC_MSAP_SCRATCHPAD_CLEAR_REQ
@ WAPS_FUNC_MSAP_SCAN_NBORS_IND
@ WAPS_FUNC_MSAP_APP_CONFIG_WRITE_REQ
@ WAPS_FUNC_MSAP_STACK_SLEEP_STATE_GET_REQ
@ WAPS_FUNC_MSAP_ATTR_WRITE_REQ
@ WAPS_FUNC_MSAP_STACK_SLEEP_STOP_CNF
bool WapsFunc_isConfirmation(uint8_t func)
Check if given func code is a confirmation.
@ WAPS_FUNC_MSAP_SCRATCHPAD_CLEAR_CNF
@ WAPS_FUNC_MSAP_STACK_SLEEP_GOTOSLEEPINFO_RSP
@ WAPS_FUNC_MSAP_STACK_STOP_CNF
@ WAPS_FUNC_RESERVED_REMOTE_API_1_REQ
bool WapsFunc_isMsapRequest(uint8_t func)
Check if given func code is a MSAP request.
@ WAPS_FUNC_MSAP_SCRATCHPAD_STATUS_CNF
@ WAPS_FUNC_DSAP_DATA_TX_RSP
@ WAPS_FUNC_DSAP_DATA_RX_IND
@ WAPS_FUNC_MSAP_GET_NBORS_REQ
@ WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_READ_CNF
@ WAPS_FUNC_MSAP_APP_CONFIG_WRITE_CNF
@ WAPS_FUNC_MSAP_STACK_STATE_IND
@ WAPS_FUNC_MSAP_SCAN_NBORS_RSP
@ WAPS_FUNC_MSAP_REMOTE_STATUS_CNF
@ WAPS_FUNC_CSAP_FACTORY_RESET_CNF
@ WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_CNF
@ WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_READ_REQ
@ WAPS_FUNC_MSAP_APP_CONFIG_RX_IND
@ WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_READ_REQ
@ WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_WRITE_CNF
@ WAPS_FUNC_MSAP_STACK_SLEEP_REQ
@ WAPS_FUNC_DSAP_DATA_TX_FRAG_CNF
@ DEPRECATED_WAPS_FUNC_MSAP_MAX_MSG_QUEUEING_TIME_READ_REQ
@ WAPS_FUNC_MSAP_SCRATCHPAD_START_REQ
@ WAPS_FUNC_MSAP_STACK_START_REQ
@ WAPS_FUNC_CSAP_ATTR_WRITE_REQ
@ WAPS_FUNC_MSAP_APP_CONFIG_RX_RSP
@ WAPS_FUNC_MSAP_STACK_STOP_REQ
@ WAPS_FUNC_MSAP_STACK_SLEEP_GOTOSLEEPINFO_REQ
@ DEPRECATED_WAPS_FUNC_MSAP_MAX_MSG_QUEUEING_TIME_WRITE_REQ
@ WAPS_FUNC_MSAP_APP_CONFIG_READ_REQ
@ WAPS_FUNC_MSAP_SCRATCHPAD_STATUS_REQ
@ WAPS_FUNC_DSAP_DATA_TX_TT_CNF
@ WAPS_FUNC_DSAP_DATA_TX_IND
@ WAPS_FUNC_MSAP_STACK_SLEEP_REQ_CNF
@ WAPS_FUNC_DSAP_DATA_RX_RSP
@ WAPS_FUNC_MSAP_GET_NBORS_CNF
@ WAPS_FUNC_RESERVED_REMOTE_API_1_CNF
@ WAPS_FUNC_MSAP_INDICATION_POLL_CNF
@ WAPS_FUNC_MSAP_SCRATCHPAD_START_CNF
@ WAPS_FUNC_MSAP_ATTR_READ_CNF
bool WapsFunc_isDsapRequest(uint8_t func)
Check if given func code is a DSAP request.
bool WapsFunc_isResponse(uint8_t func)
Check if given func code is a response.
@ WAPS_FUNC_MSAP_SINK_COST_READ_REQ
bool WapsFunc_isIndication(uint8_t func)
Check if given func code is an indication.
@ WAPS_FUNC_RESERVED_REMOTE_API_2_REQ
@ WAPS_FUNC_MSAP_SCRATCHPAD_BOOTABLE_REQ
@ DEPRECATED_WAPS_FUNC_MSAP_MAX_MSG_QUEUEING_TIME_READ_CNF
@ WAPS_FUNC_MSAP_STACK_SLEEP_STOP_REQ
@ WAPS_FUNC_MSAP_STACK_START_CNF
@ WAPS_FUNC_MSAP_REMOTE_STATUS_REQ
@ WAPS_FUNC_MSAP_REMOTE_STATUS_RSP
@ WAPS_FUNC_MSAP_GET_INSTALL_QUALITY_REQ
@ WAPS_FUNC_MSAP_SINK_COST_WRITE_CNF
@ WAPS_FUNC_CSAP_ATTR_READ_CNF
@ WAPS_FUNC_CSAP_FACTORY_RESET_REQ
bool WapsFunc_isCsapRequest(uint8_t func)
Check if given func code is a CSAP request.
@ WAPS_FUNC_MSAP_INDICATION_POLL_REQ
@ WAPS_FUNC_RESERVED_REMOTE_API_2_CNF
@ WAPS_FUNC_MSAP_STACK_STATE_RSP
@ WAPS_FUNC_RESERVED_REMOTE_API_3_CNF
@ WAPS_FUNC_MSAP_SCRATCHPAD_TARGET_READ_CNF
@ WAPS_FUNC_MSAP_SINK_COST_READ_CNF
@ WAPS_FUNC_MSAP_ATTR_WRITE_CNF
@ WAPS_FUNC_DSAP_DATA_TX_TT_REQ
@ WAPS_FUNC_DSAP_DATA_RX_FRAG_RSP
@ WAPS_FUNC_MSAP_SCRATCHPAD_BLOCK_REQ
@ WAPS_FUNC_MSAP_SINK_COST_WRITE_REQ
@ WAPS_FUNC_RESERVED_REMOTE_API_4_CNF
@ WAPS_FUNC_RESERVED_REMOTE_API_3_REQ
@ WAPS_FUNC_MSAP_SCAN_NBORS_CNF
@ WAPS_FUNC_MSAP_ATTR_READ_REQ
@ DEPRECATED_WAPS_FUNC_MSAP_MAX_MSG_QUEUEING_TIME_WRITE_CNF
@ WAPS_FUNC_MSAP_GET_INSTALL_QUALITY_CNF
@ WAPS_FUNC_CSAP_ATTR_WRITE_CNF
@ WAPS_FUNC_MSAP_SCRATCHPAD_BOOTABLE_CNF
@ WAPS_FUNC_MSAP_REMOTE_STATUS_IND
@ DEPRECATED_WAPS_FUNC_MSAP_REMOTE_UPDATE_CNF
@ WAPS_FUNC_CSAP_ATTR_READ_REQ
bool WapsFunc_isRequest(uint8_t func)
Check if given func code is a request.