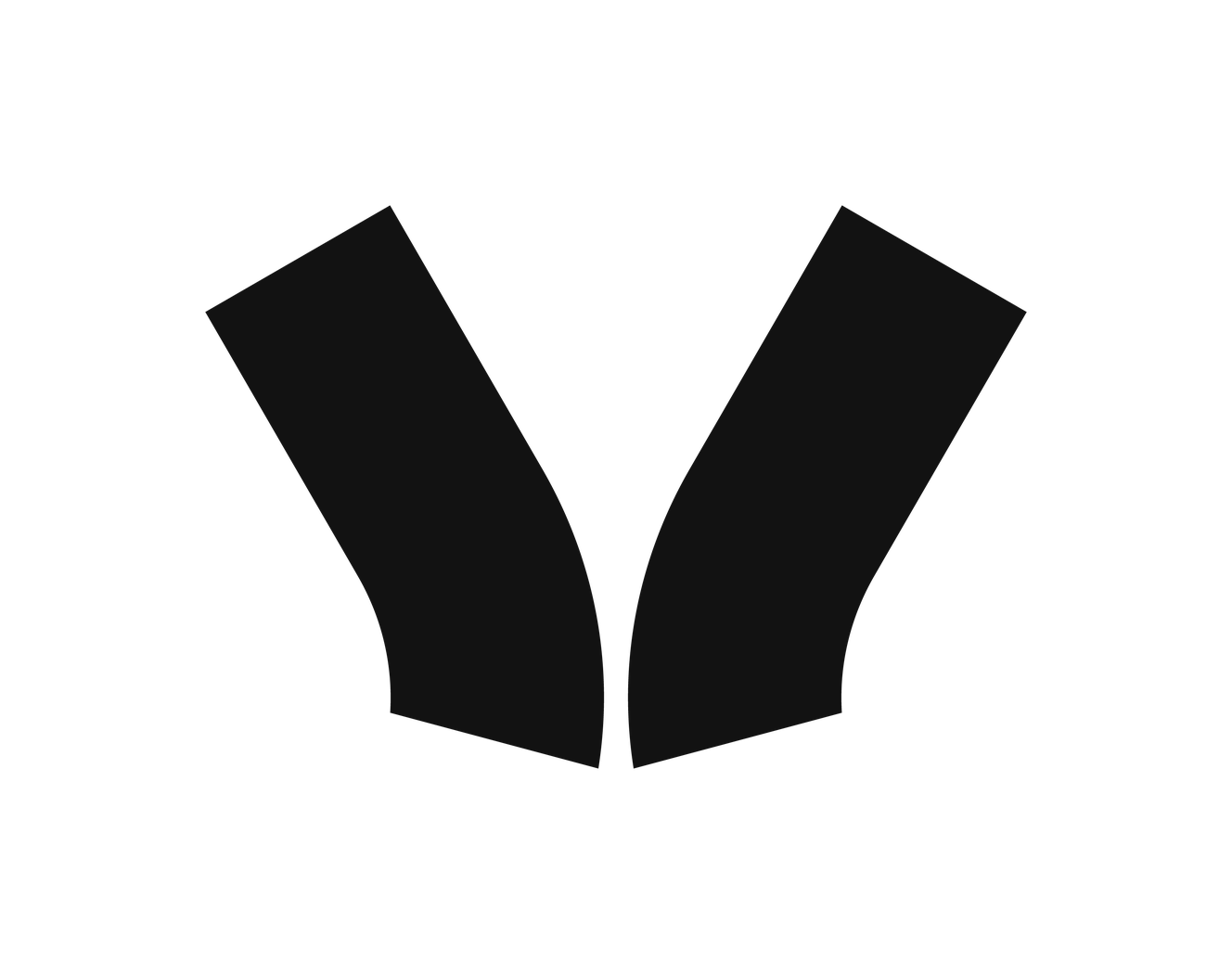 |
Wirepas SDK
|
|
Go to the documentation of this file.
39 #define TINYCBOR_VERSION ((TINYCBOR_VERSION_MAJOR << 16) | (TINYCBOR_VERSION_MINOR << 8) | TINYCBOR_VERSION_PATCH)
55 # define SIZE_MAX ((size_t)-1)
61 #ifndef CBOR_PRIVATE_API
62 # define CBOR_PRIVATE_API
64 #ifndef CBOR_INLINE_API
65 # if defined(__cplusplus)
66 # define CBOR_INLINE inline
67 # define CBOR_INLINE_API inline
69 # define CBOR_INLINE_API static CBOR_INLINE
70 # if defined(_MSC_VER)
71 # define CBOR_INLINE __inline
72 # elif defined(__GNUC__)
73 # define CBOR_INLINE __inline__
74 # elif defined(__STDC_VERSION__) && __STDC_VERSION__ >= 199901L
75 # define CBOR_INLINE inline
127 #define CborDateTimeStringTag CborDateTimeStringTag
128 #define CborUnixTime_tTag CborUnixTime_tTag
129 #define CborPositiveBignumTag CborPositiveBignumTag
130 #define CborNegativeBignumTag CborNegativeBignumTag
131 #define CborDecimalTag CborDecimalTag
132 #define CborBigfloatTag CborBigfloatTag
133 #define CborCOSE_Encrypt0Tag CborCOSE_Encrypt0Tag
134 #define CborCOSE_Mac0Tag CborCOSE_Mac0Tag
135 #define CborCOSE_Sign1Tag CborCOSE_Sign1Tag
136 #define CborExpectedBase64urlTag CborExpectedBase64urlTag
137 #define CborExpectedBase64Tag CborExpectedBase64Tag
138 #define CborExpectedBase16Tag CborExpectedBase16Tag
139 #define CborEncodedCborTag CborEncodedCborTag
140 #define CborUrlTag CborUrlTag
141 #define CborBase64urlTag CborBase64urlTag
142 #define CborBase64Tag CborBase64Tag
143 #define CborRegularExpressionTag CborRegularExpressionTag
144 #define CborMimeMessageTag CborMimeMessageTag
145 #define CborCOSE_EncryptTag CborCOSE_EncryptTag
146 #define CborCOSE_MacTag CborCOSE_MacTag
147 #define CborCOSE_SignTag CborCOSE_SignTag
148 #define CborSignatureTag CborSignatureTag
209 ptrdiff_t bytes_needed;
252 return encoder->
data.ptr;
257 return (
size_t)(encoder->
data.ptr - buffer);
262 return encoder->
end ? 0 : (size_t)encoder->
data.bytes_needed;
333 *result = !!value->
extra;
343 *result = (uint8_t)value->
extra;
374 *result = -*result - 1;
383 *result = -*result - 1;
505 memcpy(result, &data,
sizeof(*result));
517 memcpy(result, &data,
sizeof(*result));
584 __attribute__((__format__(printf, 2, 3)))
591 #if !defined(__STDC_HOSTED__) || __STDC_HOSTED__-0 == 1
@ CborErrorOverlongEncoding
static CborType cbor_value_get_type(const CborValue *value)
static bool cbor_value_is_double(const CborValue *value)
#define CborExpectedBase64urlTag
#define CborCOSE_EncryptTag
static bool cbor_value_is_boolean(const CborValue *value)
@ CborPrettyIndicateIndeterminateLength
static CborError cbor_encode_float(CborEncoder *encoder, float value)
@ CborValidateNoUnknownSimpleTypes
@ CborPrettyIndicateOverlongNumbers
static CborError cbor_value_get_array_length(const CborValue *value, size_t *length)
static CborError cbor_encode_double(CborEncoder *encoder, double value)
static bool cbor_value_is_unsigned_integer(const CborValue *value)
@ CborIteratorFlag_UnknownLength
@ CborErrorInappropriateTagForType
static CborError cbor_encode_undefined(CborEncoder *encoder)
#define CborMimeMessageTag
@ CborValidateShortestIntegrals
@ CborErrorUnknownSimpleType
@ CborValidateNoIndeterminateLength
CBOR_API CborError cbor_encode_floating_point(CborEncoder *encoder, CborType fpType, const void *value)
static CborError cbor_value_to_pretty(FILE *out, const CborValue *value)
static size_t cbor_encoder_get_buffer_size(const CborEncoder *encoder, const uint8_t *buffer)
CBOR_API CborError cbor_value_to_pretty_advance_flags(FILE *out, CborValue *value, int flags)
@ CborValidateMapIsSorted
static bool cbor_value_is_simple_type(const CborValue *value)
uint64_t _cbor_value_decode_int64_internal(const CborValue *value)
@ CborValidateMapKeysAreUnique
CBOR_API CborError cbor_value_validate(const CborValue *it, uint32_t flags)
CBOR_API CborError cbor_value_calculate_string_length(const CborValue *value, size_t *length)
#define CborUnixTime_tTag
static bool cbor_value_is_valid(const CborValue *value)
static CborError cbor_value_get_raw_integer(const CborValue *value, uint64_t *result)
@ CborErrorJsonNotImplemented
CBOR_API CborError cbor_value_skip_tag(CborValue *it)
CBOR_API CborError cbor_encode_byte_string(CborEncoder *encoder, const uint8_t *string, size_t length)
@ CborIteratorFlag_NegativeInteger
#define CborPositiveBignumTag
static CborError cbor_value_get_simple_type(const CborValue *value, uint8_t *result)
static CborError cbor_encode_half_float(CborEncoder *encoder, const void *value)
CBOR_API CborError cbor_encode_int(CborEncoder *encoder, int64_t value)
static bool cbor_value_is_undefined(const CborValue *value)
CBOR_API CborError cbor_value_to_pretty_advance(FILE *out, CborValue *value)
@ CborValidateMapKeysAreString
CborError _cbor_value_copy_string(const CborValue *value, void *buffer, size_t *buflen, CborValue *next)
union CborEncoder::@10 data
@ CborErrorJsonObjectKeyIsAggregate
@ CborValidateCompleteData
#define CborDateTimeStringTag
static CborError cbor_value_get_int64(const CborValue *value, int64_t *result)
CBOR_API CborError cbor_value_to_pretty_stream(CborStreamFunction streamFunction, void *token, CborValue *value, int flags)
static CborError cbor_value_get_double(const CborValue *value, double *result)
static bool cbor_value_is_container(const CborValue *it)
CBOR_API CborError cbor_value_text_string_equals(const CborValue *value, const char *string, bool *result)
@ CborValidateNoUnknownTags
CBOR_API CborError cbor_value_enter_container(const CborValue *it, CborValue *recursed)
#define CborEncodedCborTag
@ CborPrettyMergeStringFragments
@ CborPrettyIndicateIndetermineLength
static CborError cbor_value_copy_byte_string(const CborValue *value, uint8_t *buffer, size_t *buflen, CborValue *next)
static bool cbor_value_is_map(const CborValue *value)
static CborError cbor_value_get_map_length(const CborValue *value, size_t *length)
@ CborValidateNoUnknownSimpleTypesSA
CBOR_API CborError cbor_parser_init(const uint8_t *buffer, size_t size, uint32_t flags, CborParser *parser, CborValue *it)
CBOR_API CborError cbor_encoder_close_container_checked(CborEncoder *encoder, const CborEncoder *containerEncoder)
#define CborNegativeBignumTag
@ CborErrorUnexpectedBreak
CBOR_API CborError cbor_value_advance_fixed(CborValue *it)
static CborError cbor_value_dup_text_string(const CborValue *value, char **buffer, size_t *buflen, CborValue *next)
static size_t cbor_encoder_get_extra_bytes_needed(const CborEncoder *encoder)
@ CborErrorMapKeysNotUnique
CBOR_API void cbor_encoder_init(CborEncoder *encoder, uint8_t *buffer, size_t size, int flags)
static bool cbor_value_is_array(const CborValue *value)
static CborError cbor_value_dup_byte_string(const CborValue *value, uint8_t **buffer, size_t *buflen, CborValue *next)
static CborError cbor_value_get_boolean(const CborValue *value, bool *result)
static uint8_t * _cbor_encoder_get_buffer_pointer(const CborEncoder *encoder)
CBOR_API CborError cbor_value_get_half_float(const CborValue *value, void *result)
static bool cbor_value_is_byte_string(const CborValue *value)
static bool cbor_value_is_negative_integer(const CborValue *value)
@ CborValidateShortestFloatingPoint
@ CborIteratorFlag_IteratingStringChunks
static bool cbor_value_is_integer(const CborValue *value)
static CborError cbor_value_get_float(const CborValue *value, float *result)
CborError(* CborStreamFunction)(void *token, const char *fmt,...)
@ CborErrorNestingTooDeep
@ CborErrorDuplicateObjectKeys
static CborError cbor_encode_boolean(CborEncoder *encoder, bool value)
const CborParser * parser
static const uint8_t * cbor_value_get_next_byte(const CborValue *it)
CBOR_API CborError cbor_value_advance(CborValue *it)
@ CborValidateNoUnknownTagsSA
static CborError cbor_encode_null(CborEncoder *encoder)
@ CborIteratorFlag_ContainerIsMap
@ CborPrettyTextualEncodingIndicators
static uint64_t _cbor_value_extract_int64_helper(const CborValue *value)
CBOR_API CborError cbor_encode_simple_value(CborEncoder *encoder, uint8_t value)
@ CborPrettyShowStringFragments
static bool cbor_value_is_tag(const CborValue *value)
CBOR_API CborError cbor_encode_uint(CborEncoder *encoder, uint64_t value)
CBOR_API CborError cbor_value_leave_container(CborValue *it, const CborValue *recursed)
const CBOR_API char * cbor_error_string(CborError error)
@ CborErrorInvalidUtf8TextString
@ CborValidateNoUndefined
static CborError cbor_value_copy_text_string(const CborValue *value, char *buffer, size_t *buflen, CborValue *next)
@ CborErrorJsonObjectKeyNotString
@ CborValidateShortestNumbers
@ CborValidateCanonicalFormat
@ CborErrorIllegalSimpleType
CBOR_API CborError cbor_encode_tag(CborEncoder *encoder, CborTag tag)
@ CborErrorAdvancePastEOF
static bool cbor_value_is_half_float(const CborValue *value)
#define CborExpectedBase16Tag
@ CborErrorMapKeyNotString
CBOR_API CborError cbor_value_get_int_checked(const CborValue *value, int *result)
CBOR_API CborError cbor_encoder_create_map(CborEncoder *encoder, CborEncoder *mapEncoder, size_t length)
CBOR_API CborError cbor_encode_negative_int(CborEncoder *encoder, uint64_t absolute_value)
static const size_t CborIndefiniteLength
#define CborExpectedBase64Tag
static CborError cbor_value_get_int(const CborValue *value, int *result)
CborError _cbor_value_dup_string(const CborValue *value, void **buffer, size_t *buflen, CborValue *next)
static CborError cbor_value_get_uint64(const CborValue *value, uint64_t *result)
CBOR_API CborError cbor_encoder_create_array(CborEncoder *encoder, CborEncoder *arrayEncoder, size_t length)
@ CborValidateFiniteFloatingPoint
static bool cbor_value_at_end(const CborValue *it)
#define CborCOSE_Sign1Tag
static bool cbor_value_is_length_known(const CborValue *value)
static CborError cbor_encode_text_stringz(CborEncoder *encoder, const char *string)
#define CborRegularExpressionTag
CBOR_API CborError cbor_encoder_close_container(CborEncoder *encoder, const CborEncoder *containerEncoder)
CBOR_API CborError cbor_value_get_int64_checked(const CborValue *value, int64_t *result)
@ CborPrettyNumericEncodingIndicators
CBOR_API CborError cbor_encode_text_string(CborEncoder *encoder, const char *string, size_t length)
@ CborValidateNoUnknownTagsSR
@ CborErrorUnsupportedType
static CborError cbor_value_get_tag(const CborValue *value, CborTag *result)
#define CborCOSE_Encrypt0Tag
static bool cbor_value_is_text_string(const CborValue *value)
static bool cbor_value_is_float(const CborValue *value)
CBOR_API CborError cbor_value_validate_basic(const CborValue *it)
@ CborIteratorFlag_IntegerValueTooLarge
static bool cbor_value_is_null(const CborValue *value)
CBOR_API CborError cbor_value_map_find_value(const CborValue *map, const char *string, CborValue *element)
static CborError cbor_value_get_string_length(const CborValue *value, size_t *length)