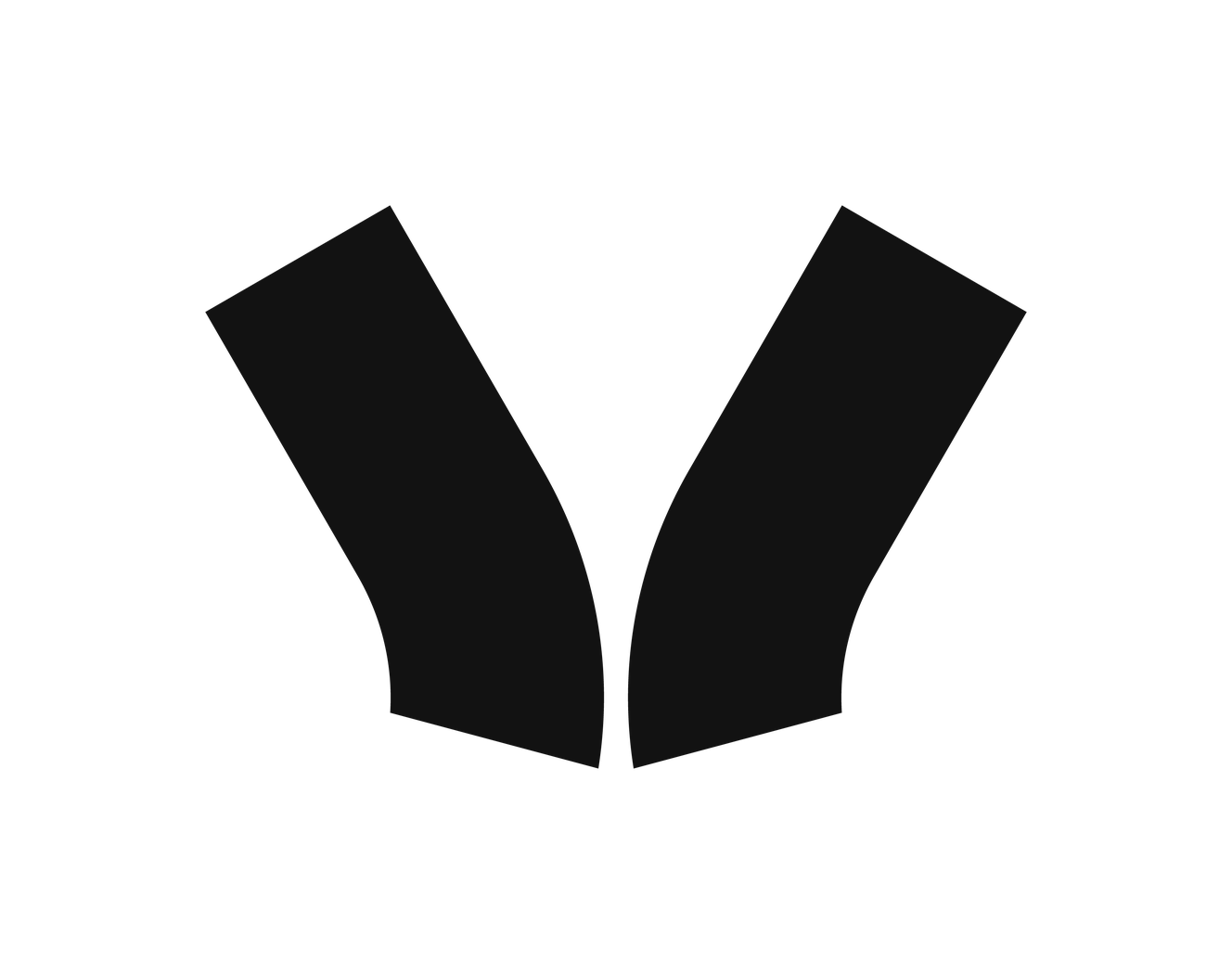 |
Wirepas SDK
|
|
Go to the documentation of this file.
13 #define AES_128_KEY_BLOCK_SIZE 16
67 const uint8_t * key128_ptr,
68 const uint8_t * iv_ctr_ptr);
92 uint8_t * mic_out_ptr,
93 uint_fast8_t mic_out_bytes,
94 const uint8_t * intext_ptr,
111 const uint8_t * intext_ptr,
112 uint8_t * outtext_ptr,
AES-128 key, input data and output data.
void aes_omac1(aes_omac1_state_t *state, uint8_t *mic_out_ptr, uint_fast8_t mic_out_bytes, const uint8_t *intext_ptr, size_t intext_bytes)
Calculate and write out OMAC1 (CMAC) MIC for input text.
For storing 128bit keys, IVs, MACs etc. Allows both byte and faster 32-bit word-aligned access.
void aes_crypto128Ctr(aes_data_stream_t *stream_ptr, const uint8_t *intext_ptr, uint8_t *outtext_ptr, size_t bytecount)
Run the AES128 cryptography in CTR mode.
void aes_setupStream(aes_data_stream_t *stream_ptr, const uint8_t *key128_ptr, const uint8_t *iv_ctr_ptr)
Setup generic AES128 CTR mode stream.
void aes_initOmac1(aes_omac1_state_t *state_ptr, const uint8_t *mic_key_ptr)
Initializes an OMAC1 state.