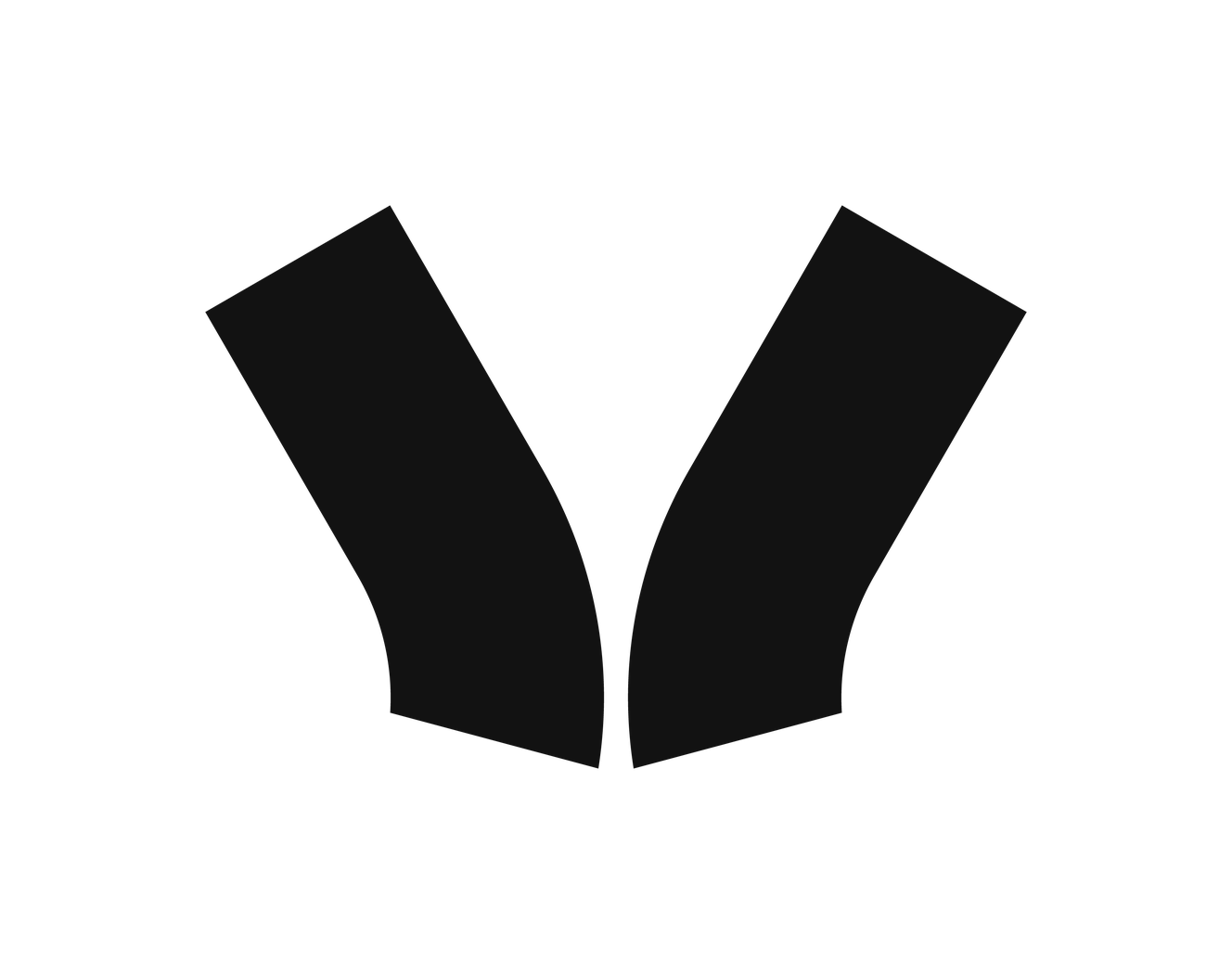 |
Wirepas SDK
|
|
Go to the documentation of this file.
20 #define __STATIC_INLINE static inline
28 #define APP_API_VERSION 0x200
31 #define APP_V2_TAG ("APP2\171\306\073\165" \
32 "\263\303\334\322\035\266\006\115")
35 #define APP_V2_TAG_LENGTH 16
38 #define APP_V2_TAG_OFFSET 48
42 #define APP_V2_TAG_MIN_API_VERSION 0x200
45 #define APP_API_V1_VERSION 0x100
152 typedef const void * (*app_open_library_f)(uint32_t name, uint32_t version);
178 const void ** reserved2,
#define APP_V2_TAG_LENGTH
app_get_api_version_f getApiVersion
uint32_t start_ram_address
static uint32_t App_getApiVersion(const void *const global_cb)
app_open_library_f openLibrary
@ APP_RES_RESOURCE_UNAVAILABLE
intptr_t App_entrypoint(const void *functions, size_t reserved1, const void **reserved2, void **ram_top)
Application initial entrypoint.
@ APP_ADDR_BROADCAST
Send packet as broadcast to all nodes.
app_firmware_version_t(* app_get_stack_firmware_version_f)(void)
Get stack firmware version.
@ APP_RES_NOT_IMPLEMENTED
#define APP_API_V1_VERSION
@ APP_RES_UNSPECIFIED_ERROR
@ APP_RES_INVALID_CONFIGURATION
List of global functions, passed to App_entrypoint()
uint32_t(* get_api_version_compatible_f)(void)
@ APP_ADDR_MULTICAST_LAST
app_special_addr_e
Special destination addresses for sending packets.
@ APP_RES_INVALID_NULL_POINTER
app_get_stack_firmware_version_f getStackFirmwareVersion
uint32_t(* app_get_api_version_f)(void)
@ APP_RES_INVALID_STACK_STATE
const typedef void *(* app_open_library_f)(uint32_t name, uint32_t version)
Open a library.