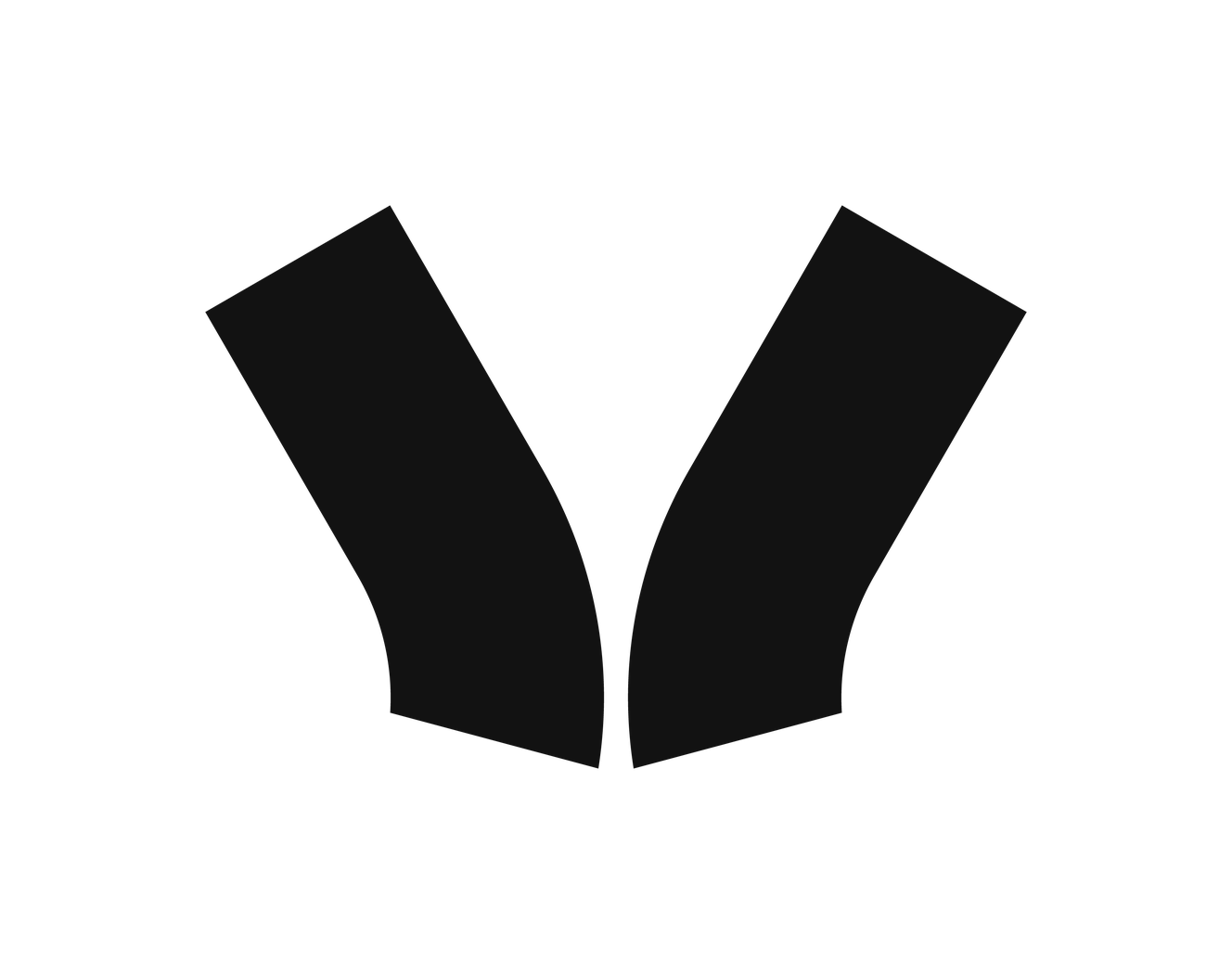 |
Wirepas SDK
|
|
Go to the documentation of this file.
70 #define sl_list_empty(l) ((l)->next == NULL)
75 #define sl_list_begin(l) sl_list_next((sl_list_t *) l)
78 #define sl_list_end(l) ((sl_list_t *) NULL)
80 #define sl_list_front(l) ((sl_list_t *)(l)->next)
83 #define SL_LIST_ITER_NEXT(iter) { (iter) = (((sl_list_t *)(iter))->next); }
125 #define sl_list_size(l) ((l)->size)
134 int (*match)(
const sl_list_t *,
const void *),
135 const void * match_param);
sl_list_t * sl_list_next(const sl_list_t *iter)
void sl_list_swap(sl_list_head_t *list1, sl_list_head_t *list2)
void sl_list_init(sl_list_head_t *list_head)
sl_list_t * sl_list_remove(sl_list_head_t *list_head, sl_list_t *element)
sl_list_t * sl_list_pop_front(sl_list_head_t *list_head)
void sl_list_push_front(sl_list_head_t *list_head, sl_list_t *element)
sl_list_t * sl_list_at(const sl_list_head_t *list_head, int idx)
sl_list_t * sl_list_pop(sl_list_head_t *list_head, sl_list_t *element)
void sl_list_push_before(sl_list_head_t *list_head, const sl_list_t *iter, sl_list_t *element)
sl_list_t * sl_list_search(const sl_list_t *start, int(*match)(const sl_list_t *, const void *), const void *match_param)
sl_list_t * sl_list_pop_back(sl_list_head_t *list_head)
void sl_list_push_back(sl_list_head_t *list_head, sl_list_t *element)
unsigned char sl_list_contains(const sl_list_head_t *list_head, const sl_list_t *element)