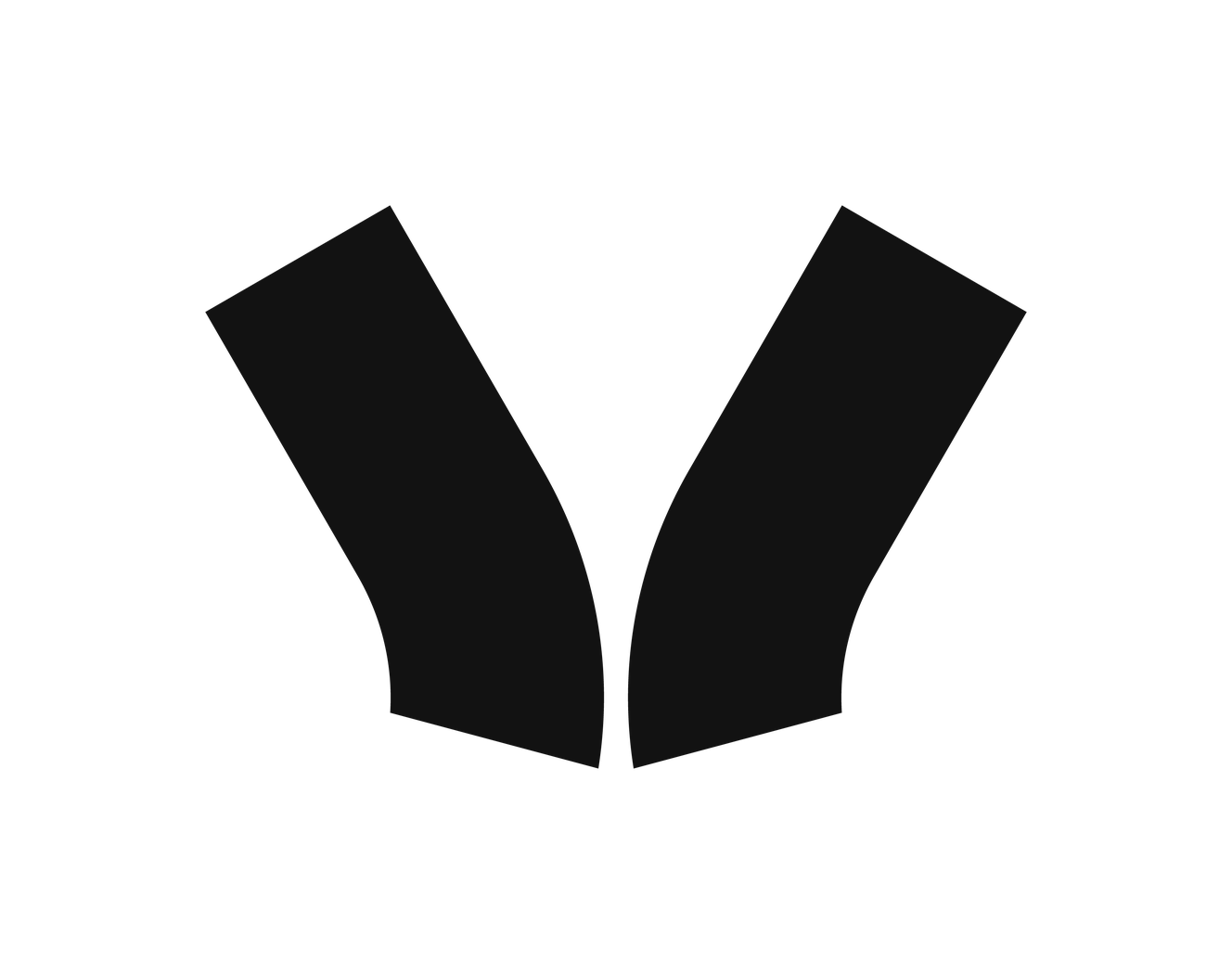 |
Wirepas SDK
|
|
Go to the documentation of this file.
6 #ifndef _PROVISIONING_H_
7 #define _PROVISIONING_H_
86 typedef const app_lib_joining_received_beacon_t *
87 (*provisioning_joining_beacon_cb_f)
88 (
const app_lib_joining_received_beacon_t * beacons);
258 #endif //_PROVISIONING_H_
const typedef app_lib_joining_received_beacon_t *(* provisioning_joining_beacon_cb_f)(const app_lib_joining_received_beacon_t *beacons)
Selects which joining beacon to connect to at the end of a scan.
This structure holds the joining proxy parameters.
provisioning_res_e
Provisioning result.
uint8_t app_lib_settings_net_channel_t
Network channel type definition.
@ PROV_RES_ERROR_SCANNING_BEACONS
provisioning_method_e method
@ PROV_RES_INVALID_PACKET
provisioning_ret_e Provisioning_start(void)
Start the provisioning process.
void(* provisioning_user_data_cb_f)(uint32_t id, CborType type, uint8_t *data, uint8_t len)
Received User provisioning data callback. Provisioning data is received as a map of id:data....
provisioning_ret_e Provisioning_Proxy_init(provisioning_proxy_conf_t *conf)
Initialize the provisioning proxy.
provisioning_ret_e Provisioning_Proxy_start(void)
Start sending joining beacons. Provisioning packets will be forwarded to provisioning server or treat...
provisioning_ret_e Provisioning_init(provisioning_conf_t *conf)
Initialize the provisioning process.
@ PROV_RES_ERROR_SENDING_DATA
This structure holds the provisioning parameters.
@ PROV_RET_JOINING_LIB_ERROR
uint32_t app_lib_settings_net_addr_t
Network address type definition.
app_lib_settings_net_channel_t net_chan
provisioning_ret_e Provisioning_stop(void)
Stops the provisioning process.
@ PROV_RET_INTERNAL_ERROR
provisioning_method_e
supported provisioning methods
bool is_local_unsec_allowed
provisioning_ret_e
Return codes of provisioning functions.
provisioning_ret_e Provisioning_Proxy_stop(void)
Stops the provisioning proxy.
provisioning_joining_beacon_cb_f beacon_joining_cb
#define APP_LIB_SETTINGS_AES_KEY_NUM_BYTES
AES key size in bytes.
bool(* provisioning_end_cb_f)(provisioning_res_e result)
The end provisioning callback. This function is called at the end of the provisioning process.
bool(* provisioning_proxy_start_cb_f)(const uint8_t *uid, uint8_t uid_len, provisioning_method_e method, provisioning_proxy_net_param_t *net_param)
The proxy received START packet callback. This function is called when the proxy receives a valid STA...
provisioning_user_data_cb_f user_data_cb
provisioning_proxy_start_cb_f start_cb
@ PROV_RES_ERROR_NO_ROUTE
@ PROV_RES_ERROR_INTERNAL
bool is_local_sec_allowed
This structure contains the network parameters sent by the provisioning proxy to the new node.
@ PROV_METHOD_EXTENDED_UID
provisioning_end_cb_f end_cb
app_lib_settings_net_addr_t net_addr