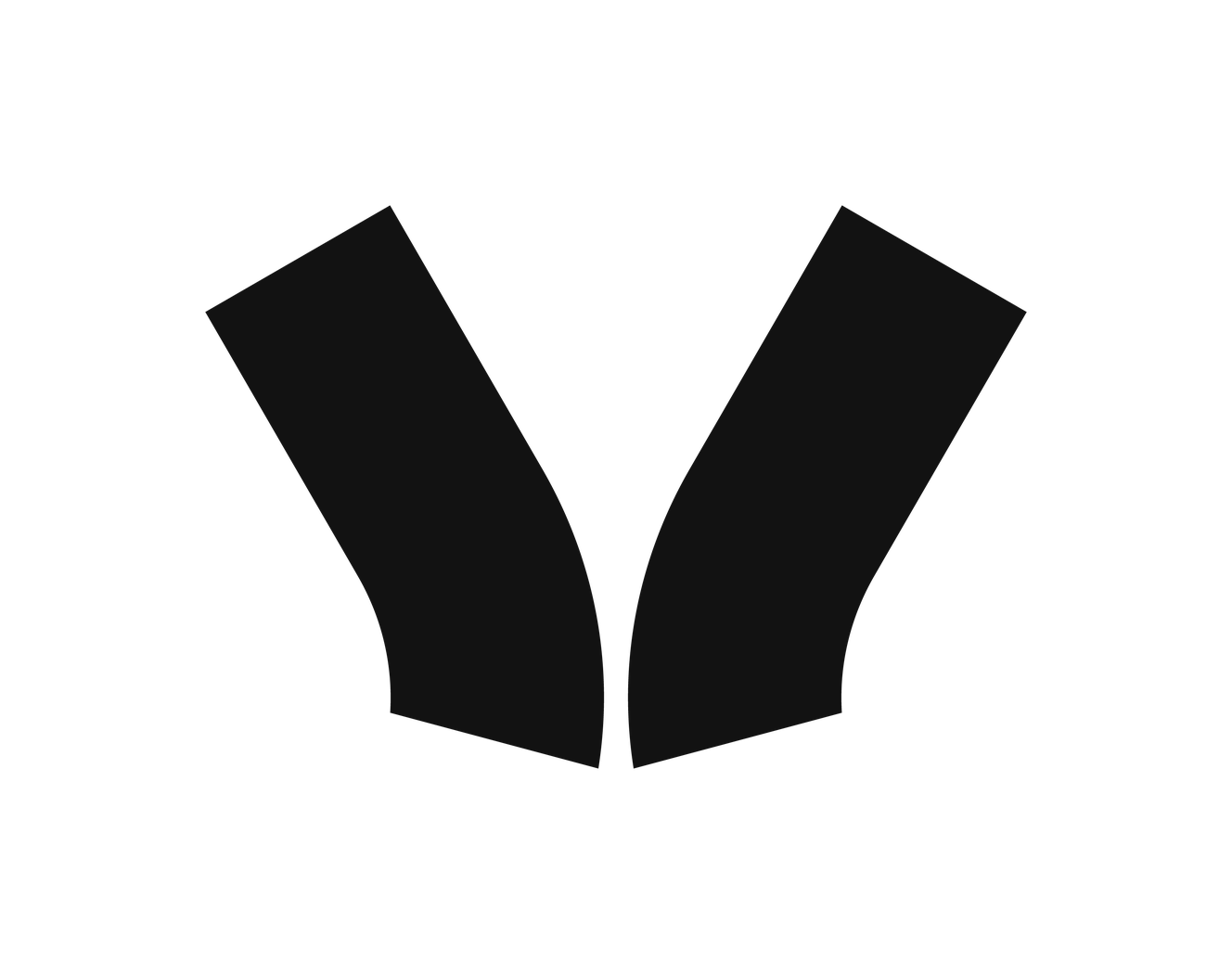 |
Wirepas SDK
|
|
Go to the documentation of this file.
15 #ifndef APP_LIB_STATE_H_
16 #define APP_LIB_STATE_H_
27 #define APP_LIB_STATE_NAME 0x02f9c165
30 #define APP_LIB_STATE_VERSION 0x20D
37 #define APP_LIB_STATE_INVALID_ROUTE_COST UINT8_MAX
44 #define APP_LIB_STATE_COST_UNKNOWN 0
51 #define APP_LIB_STATE_LINKREL_UNKNOWN 0
@ APP_LIB_STATE_NETWORK_ADDRESS_NOT_SET
Network address is not set.
app_res_e(* app_lib_state_start_scan_nbors_f)(void)
Start neighbor scan.
app_lib_state_get_route_f getRouteInfo
@ APP_LIB_STATE_ROLE_NOT_SET
Node role is not set.
app_lib_settings_net_channel_t channel
app_lib_state_scan_type_e scan_type
Installation quality information. Contains information about the nodes installation location i....
uint8_t app_lib_settings_net_channel_t
Network channel type definition.
bool is_sink
Device is sink.
app_res_e(* app_lib_state_scan_stop_f)(void)
Stops ongoing scan operation before ended.
app_res_e(* app_lib_state_get_sink_cost_f)(uint8_t *cost_p)
Query the currently set additional penalty for the sink usage.
app_lib_state_beacon_type_e
Type for beacons, passed in app_lib_state_beacon_rx_t.
@ APP_LIB_STATE_NEIGHBOR_IS_CLUSTER
app_lib_state_get_access_cycle_f getAccessCycle
app_res_e(* app_lib_state_set_stack_event_cb_f)(app_lib_state_on_stack_event_cb_f cb)
Register callback for stack events.
app_res_e(* app_lib_state_get_install_quality_f)(app_lib_state_install_quality_t *qual_out)
Read installation quality, app_lib_state_install_quality_t.
app_lib_state_get_stack_state_f getStackState
int8_t txpower
Tx power in dB This equals maximum transmission power that sender can transmit (which is used when tr...
Structure to hold the information about received beacons.
@ APP_LIB_STATE_SCAN_NBORS_ALL
@ APP_LIB_STATE_STACK_EVENT_SCRAT_XFER_STARTED
@ APP_LIB_STATE_INSTALL_QUALITY_ERROR_NOROUTE
@ APP_LIB_STATE_STACK_EVENT_STACK_STARTED
void(* app_lib_state_on_stack_event_cb_f)(app_lib_stack_event_e event, void *param)
Function type stack events callback.
app_res_e(* app_lib_state_get_access_cycle_f)(uint16_t *ac_value_p)
Get current access cycle.
app_lib_state_nbor_info_t * nbors
@ APP_LIB_STATE_STACK_EVENT_SCRAT_XFER_STOPPED
app_lib_stack_event_e
List of event generated by the stack when registering to app_lib_state_set_stack_event_cb_f.
bool is_da_support
Sender supports Directed Advertiser sending packets to it.
Structure for route information.
app_res_e(* app_lib_state_set_on_beacon_cb_f)(app_lib_state_on_beacon_cb_f cb)
Set a callback to be called when a beacon is received.
app_lib_state_route_state_e state
@ APP_LIB_STATE_STOPPED
Stack is stopped.
app_lib_state_scan_type_e
Types of scans.
void(* app_lib_state_on_beacon_cb_f)(const app_lib_state_beacon_rx_t *beacon)
Function type for a beacon reception callback.
app_res_e(* app_lib_state_start_stack_f)(void)
Start the stack.
List of library functions.
@ APP_LIB_STATE_NEIGHBOR_IS_NEXT_HOP
@ APP_LIB_STATE_DIRADV_SUPPORTED
@ APP_LIB_STATE_DIRADV_NOT_SUPPORTED
@ APP_LIB_STATE_ACCESS_DENIED
Operation is not allowed.
app_lib_state_scan_stop_f stopScanNbors
app_lib_state_diradv_support_e
Support type for directed advertiser, i.e. does neighbor support sending directed advertiser packets ...
app_lib_state_start_stack_f startStack
app_lib_state_start_scan_nbors_f startScanNbors
app_lib_state_scan_nbors_type_e
Scan neighbor type to specify the scans that trigger the callback.
@ APP_LIB_STATE_STARTED
Stack is started.
@ SCAN_TYPE_APP_ORIGINATED
@ APP_LIB_STATE_INSTALL_QUALITY_ERROR_NONBORS
Neighbors list definition.
install_quality_error_code_e
Error codes for installation quality, if an error code is active, corrective action regarding the ins...
app_addr_t address
Address of the beacon sender.
@ APP_LIB_STATE_NODE_ADDRESS_NOT_SET
Node address is not set.
uint8_t(* app_lib_state_get_stack_state_f)(void)
Get the stack state.
app_res_e(* app_lib_state_get_nbors_f)(app_lib_state_nbor_list_t *nbors_list)
Get list of neighbors.
app_lib_state_route_state_e
Route state.
Information on neighbor scan.
app_res_e(* app_lib_state_stop_stack_f)(void)
Stop the stack.
@ APP_LIB_STATE_DIRADV_UNKNOWN
app_lib_state_stop_stack_f stopStack
app_res_e(* app_lib_state_set_sink_cost_f)(const uint8_t cost)
Set additional penalty for the sink usage.
@ APP_LIB_STATE_ROUTE_STATE_INVALID
No next hop / route.
app_lib_state_nbor_type_e
Neighbor type.
@ APP_LIB_STATE_APP_CONFIG_DATA_NOT_SET
@ APP_LIB_STATE_SCAN_NBORS_ONLY_REQUESTED
Information on started scan.
@ APP_LIB_STATE_BEACON_TYPE_CB
Cluster beacon.
@ APP_LIB_STATE_ROUTE_STATE_PENDING
Acquiring next hop / route.
@ APP_LIB_STATE_NETWORK_CHANNEL_NOT_SET
Network channel is not set.
@ SCAN_TYPE_STACK_ORIGINATED
@ APP_LIB_STATE_BEACON_TYPE_NB
Network beacon.
@ APP_LIB_STATE_STACK_EVENT_SCAN_STARTED
app_lib_state_stack_state_e
Stack state flags.
Neighbors info definition.
app_lib_state_set_stack_event_cb_f setOnStackEventCb
app_lib_state_set_on_beacon_cb_f setOnBeaconCb
app_lib_state_set_sink_cost_f setSinkCost
@ APP_LIB_STATE_STACK_EVENT_STACK_STOPPED
app_lib_state_get_sink_cost_f getSinkCost
@ APP_LIB_STATE_INSTALL_QUALITY_ERROR_NONE
@ APP_LIB_STATE_STACK_EVENT_SCAN_STOPPED
@ APP_LIB_STATE_STACK_EVENT_ROUTE_CHANGED
@ APP_LIB_STATE_INSTALL_QUALITY_ERROR_BADRSSI
app_res_e(* app_lib_state_get_route_f)(app_lib_state_route_info_t *info)
Get route information.
@ APP_LIB_STATE_ROUTE_STATE_VALID
Valid next hop / route.
app_lib_state_get_install_quality_f getInstallQual
app_lib_state_get_nbors_f getNbors
@ APP_LIB_STATE_NEIGHBOR_IS_MEMBER