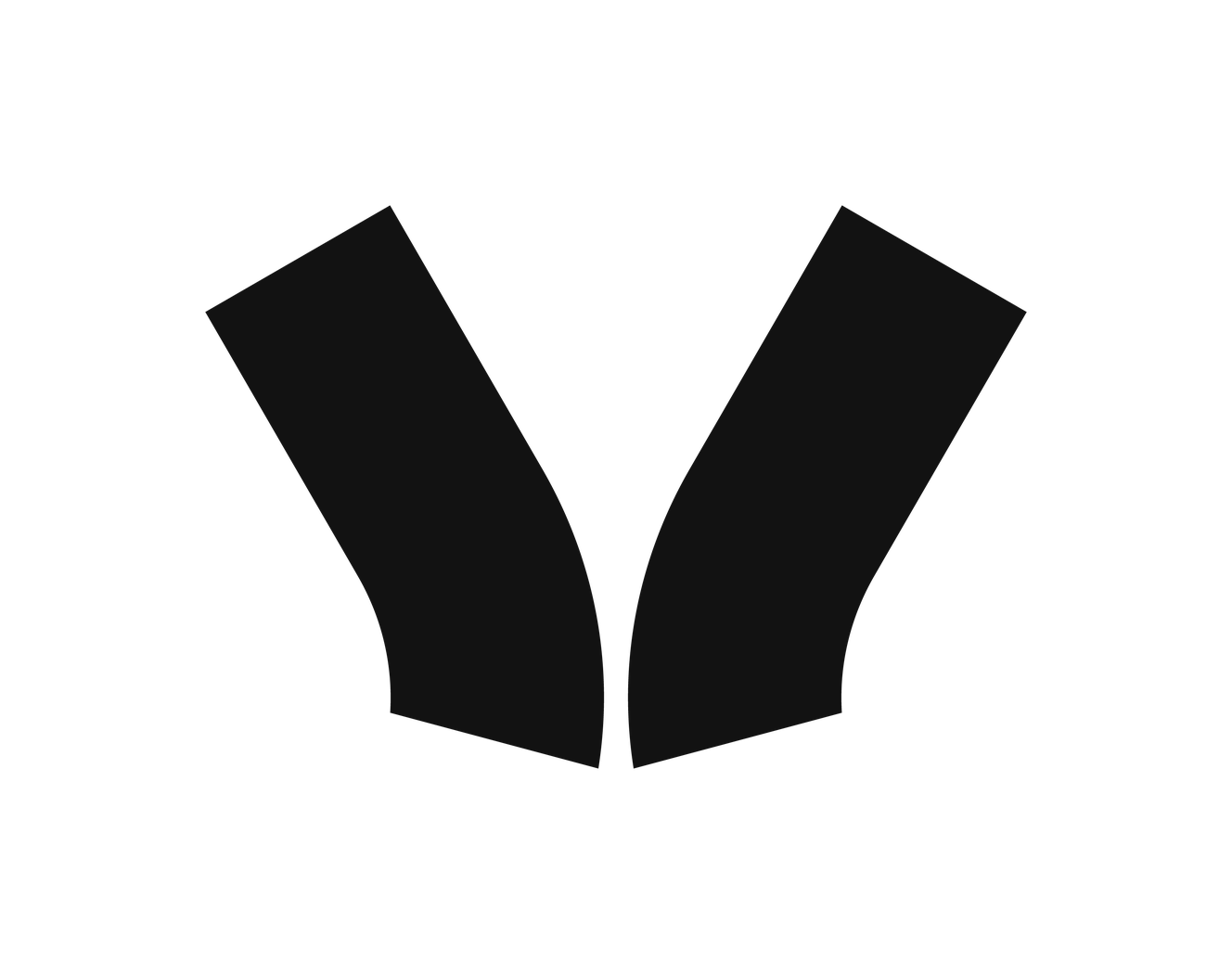 |
Wirepas SDK
|
|
Go to the documentation of this file.
20 #ifndef APP_LIB_OTAP_H_
21 #define APP_LIB_OTAP_H_
30 #define APP_LIB_OTAP_NAME 0x000f2338
33 #define APP_LIB_OTAP_VERSION 0x201
484 uint16_t * target_crc,
size_t(* app_lib_otap_get_max_block_num_bytes_f)(void)
Get maximum block size in bytes.
app_lib_otap_get_processed_num_bytes_f getProcessedNumBytes
uint8_t app_lib_otap_seq_t
Type for OTAP sequence number.
app_lib_otap_get_seq_f getSeq
size_t(* app_lib_otap_get_max_num_bytes_f)(void)
Get maximum scratchpad size.
app_lib_otap_get_processed_seq_f getProcessedSeq
app_res_e(* app_lib_otap_begin_f)(size_t num_bytes, app_lib_otap_seq_t seq)
Start writing a new scratchpad.
@ APP_LIB_OTAP_STATUS_NEW
app_lib_otap_get_num_bytes_f getNumBytes
@ APP_LIB_OTAP_TYPE_BLANK
app_res_e(* app_lib_otap_read_f)(uint32_t start, size_t num_bytes, void *bytes)
Read a block of scratchpad.
app_lib_otap_get_processed_crc_f getProcessedCrc
@ APP_LIB_OTAP_WRITE_RES_COMPLETED_ERROR
app_res_e(* app_lib_otap_set_to_be_processed_f)(void)
Mark the stored scratchpad to be eligible for processing by the bootloader.
@ APP_LIB_OTAP_WRITE_RES_NOT_ONGOING
app_lib_otap_get_processed_area_id_f getProcessedAreaId
app_res_e(* app_lib_otap_set_target_scratchpad_and_action_f)(app_lib_otap_seq_t target_sequence, uint16_t target_crc, app_lib_otap_action_e action, uint8_t delay)
This service allows to set the information for scratchpad in the sink tree. What is the target scratc...
@ APP_LIB_OTAP_TYPE_PRESENT
@ APP_LIB_OTAP_WRITE_RES_OK
app_lib_otap_get_max_num_bytes_f getMaxNumBytes
app_lib_otap_begin_f begin
app_lib_otap_clear_f clear
app_lib_otap_is_processed_f isProcessed
@ APP_LIB_OTAP_WRITE_RES_INVALID_NULL_BYTES
app_lib_otap_write_res_e
Write function return code.
size_t(* app_lib_otap_get_processed_num_bytes_f)(void)
Get the size of scratchpad, in bytes, that produced the running stack firmware.
app_lib_otap_get_target_scratchpad_and_action_f getTargetScratchpadAndAction
app_lib_otap_is_set_to_be_processed_f isSetToBeProcessed
bool(* app_lib_otap_is_valid_f)(void)
Get the scratchpad validity status.
app_lib_otap_set_to_be_processed_f setToBeProcessed
app_lib_otap_status_e(* app_lib_otap_get_status_f)(void)
Get the bootloader status of the stored scratchpad.
@ APP_LIB_OTAP_ACTION_LEGACY
app_lib_otap_get_crc_f getCrc
app_lib_otap_get_status_f getStatus
uint32_t(* app_lib_otap_get_processed_area_id_f)(void)
Get the area ID of the file in the scratchpad that produced the running stack firmware.
app_lib_otap_write_f write
app_res_e(* app_lib_otap_get_target_scratchpad_and_action_f)(app_lib_otap_seq_t *target_sequence, uint16_t *target_crc, app_lib_otap_action_e *action, uint8_t *delay)
This service allows to get the information for scratchpad in the sink tree. What is the target scratc...
app_lib_otap_type_e(* app_lib_otap_get_type_f)(void)
Get the type of the stored scratchpad.
app_lib_otap_get_max_block_num_bytes_f getMaxBlockNumBytes
uint16_t(* app_lib_otap_get_crc_f)(void)
Get the 16-bit CRC of the stored scratchpad.
app_lib_otap_is_valid_f isValid
app_lib_otap_action_e
Scratchpad possible actions.
app_lib_otap_write_res_e(* app_lib_otap_write_f)(uint32_t start, size_t num_bytes, const void *bytes)
Write a block of scratchpad.
app_lib_otap_get_type_f getType
@ APP_LIB_OTAP_WRITE_RES_INVALID_HEADER
@ APP_LIB_OTAP_WRITE_RES_INVALID_START
app_lib_otap_type_e
Different scratchpad type.
size_t(* app_lib_otap_get_num_bytes_f)(void)
Get stored scratchpad size.
@ APP_LIB_OTAP_ACTION_PROPAGATE_AND_PROCESS
uint16_t(* app_lib_otap_get_processed_crc_f)(void)
Get the 16-bit CRC of the scratchpad that produced the running stack firmware.
@ APP_LIB_OTAP_WRITE_RES_INVALID_NUM_BYTES
@ APP_LIB_OTAP_TYPE_PROCESS
app_lib_otap_status_e
Status code from the bootloader.
app_res_e(* app_lib_otap_clear_f)(void)
Erase a stored scratchpad from memory.
@ APP_LIB_OTAP_ACTION_PROPAGATE_AND_PROCESS_WITH_DELAY
bool(* app_lib_otap_is_processed_f)(void)
Check if the stored scratchpad has been processed by the bootloader.
app_lib_otap_seq_t(* app_lib_otap_get_seq_f)(void)
Get the OTAP sequence number.
@ APP_LIB_OTAP_WRITE_RES_COMPLETED_OK
@ APP_LIB_OTAP_ACTION_NO_OTAP
bool(* app_lib_otap_is_set_to_be_processed_f)(void)
Check if scratchpad is set to be processed.
app_lib_otap_seq_t(* app_lib_otap_get_processed_seq_f)(void)
Get the OTAP sequence number of the scratchpad that produced the running stack firmware.
@ APP_LIB_OTAP_ACTION_PROPAGATE_ONLY
app_lib_otap_set_target_scratchpad_and_action_f setTargetScratchpadAndAction