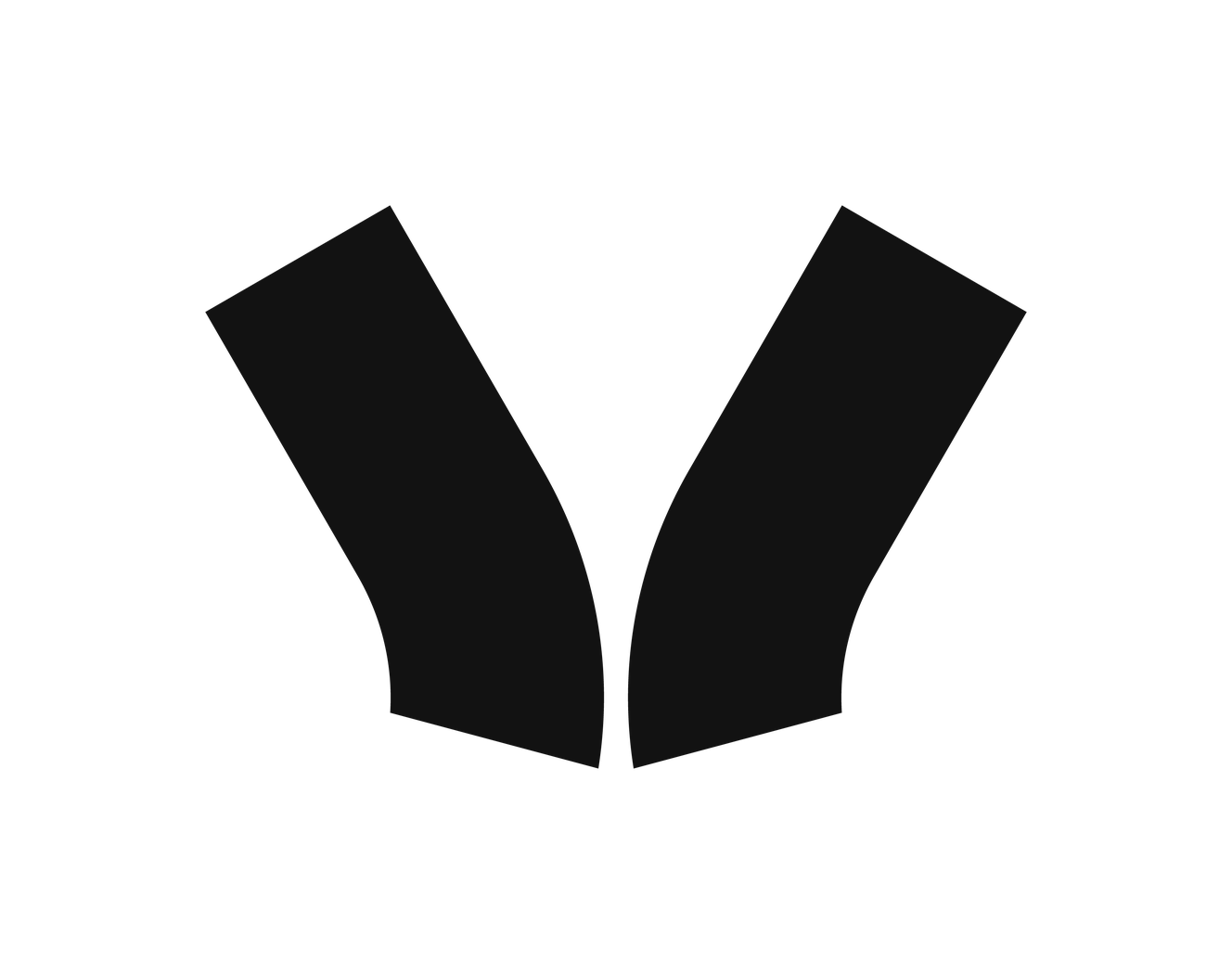 |
Wirepas SDK
|
|
Go to the documentation of this file.
90 #define ERR_NOT_OPEN APP_RES_INVALID_NULL_POINTER
93 #define Sys_enterCriticalSection() \
94 lib_system ? lib_system->enterCriticalSection() : ERR_NOT_OPEN
96 #define Sys_exitCriticalSection() \
97 lib_system ? lib_system->exitCriticalSection() : ERR_NOT_OPEN
99 #define Sys_disableDs(_dis) \
100 lib_system ? lib_system->disableDeepSleep(_dis) : ERR_NOT_OPEN
102 #define Sys_enableAppIrq(_irqn, _isr) \
103 lib_system ? lib_system->enableAppIrq(false, _irqn, APP_LIB_SYSTEM_IRQ_PRIO_LO, _isr) : ERR_NOT_OPEN
105 #define Sys_disableAppIrq(_irqn) \
106 lib_system ? lib_system->disableAppIrq(_irqn) : ERR_NOT_OPEN
108 #define Sys_enableFastAppIrq(_irqn, _prio, _isr) \
109 lib_system ? lib_system->enableAppIrq(true, _irqn, _prio, _isr) : ERR_NOT_OPEN
111 #define Sys_clearFastAppIrq(_irqn) \
112 lib_system ? lib_system->clearPendingFastAppIrq(_irqn) : ERR_NOT_OPEN
115 #define Ble_beaconRx_setRxCb(_cb) \
116 lib_beacon_rx ? lib_beacon_rx->setBeaconReceivedCb(_cb) : ERR_NOT_OPEN
118 #define Ble_beaconRx_startScanner(_chan) \
119 lib_beacon_rx ? lib_beacon_rx->startScanner(_chan) : ERR_NOT_OPEN
121 #define Ble_beaconRx_stopScanner() \
122 lib_beacon_rx ? lib_beacon_rx->stopScanner() : ERR_NOT_OPEN
124 #define Ble_beaconRx_isStarted() \
125 (lib_beacon_rx ? lib_beacon_rx->isScannerStarted() : ERR_NOT_OPEN)
List of library functions.
List of library services.
bool API_Open(const app_global_functions_t *functions)
Open API (open all libraries)
List of library functions.
List of library functions.
List of library functions.
List of library functions.
List of global functions, passed to App_entrypoint()
const app_global_functions_t *global_func __attribute((weak))
List of library functions.
List of library functions.
List of library functions.
List of library functions.
List of library functions.